设有一元多项式Am(x)和Bn(X),编程实现多项式Am(x)和Bn(x)的加法、减法和乘法运算。其中多项式描述为: Am(x)=A0+A1x1+A2x2+A3x3+….+Amxm; Bn(x)=B0+B1x1+B2x2+B3x3+….+Bnxn。
时间: 2023-11-26 19:05:24 浏览: 93
以下是Python代码实现多项式的加法、减法和乘法运算:
```python
class Polynomial:
def __init__(self, coefficients):
self.coefficients = coefficients
def __add__(self, other):
new_coefficients = []
for i in range(max(len(self.coefficients), len(other.coefficients))):
if i < len(self.coefficients) and i < len(other.coefficients):
new_coefficients.append(self.coefficients[i] + other.coefficients[i])
elif i < len(self.coefficients):
new_coefficients.append(self.coefficients[i])
else:
new_coefficients.append(other.coefficients[i])
return Polynomial(new_coefficients)
def __sub__(self, other):
new_coefficients = []
for i in range(max(len(self.coefficients), len(other.coefficients))):
if i < len(self.coefficients) and i < len(other.coefficients):
new_coefficients.append(self.coefficients[i] - other.coefficients[i])
elif i < len(self.coefficients):
new_coefficients.append(self.coefficients[i])
else:
new_coefficients.append(-other.coefficients[i])
return Polynomial(new_coefficients)
def __mul__(self, other):
new_coefficients = [0] * (len(self.coefficients) + len(other.coefficients) - 1)
for i in range(len(self.coefficients)):
for j in range(len(other.coefficients)):
new_coefficients[i+j] += self.coefficients[i] * other.coefficients[j]
return Polynomial(new_coefficients)
def __str__(self):
result = ""
for i, coefficient in enumerate(self.coefficients):
if coefficient != 0:
if i == 0:
result += str(coefficient)
elif i == 1:
result += f"{'+' if coefficient > 0 else ''}{coefficient}x"
else:
result += f"{'+' if coefficient > 0 else ''}{coefficient}x^{i}"
if result == "":
result = "0"
return result
```
其中,`__add__` 方法实现了多项式的加法运算,`__sub__` 方法实现了多项式的减法运算,`__mul__` 方法实现了多项式的乘法运算。`__str__` 方法用于将多项式对象转换为字符串。
使用示例:
```python
A = Polynomial([1, 2, 3]) # A(x) = 1 + 2x + 3x^2
B = Polynomial([4, 5]) # B(x) = 4 + 5x
C = A + B # 加法运算,C(x) = 5 + 7x + 3x^2
print(C)
D = A - B # 减法运算,D(x) = -3 - 3x + 3x^2
print(D)
E = A * B # 乘法运算,E(x) = 4 + 13x + 22x^2 + 15x^3
print(E)
```
阅读全文
相关推荐




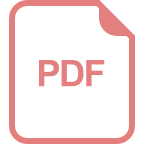
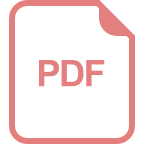
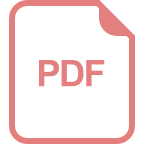
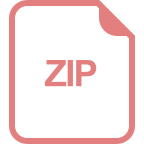
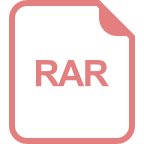
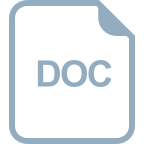
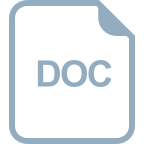
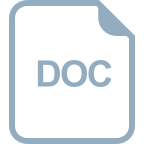
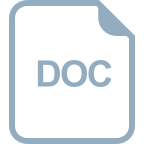
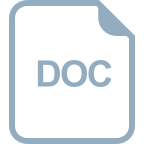
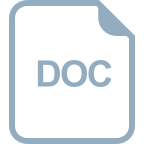