在单链表中值最大的节点后插入元素算法 用C语言实现
时间: 2024-10-21 08:06:08 浏览: 15
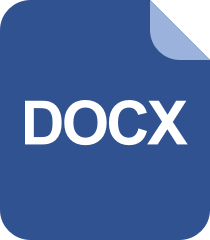
循环单链表中值为X节点删除方法
在单链表中,在值最大的节点之后插入新元素,首先需要找到链表中的最大值节点,然后将新元素添加到该节点之后。下面是一个简单的C语言函数实现这个过程:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 比较两个整数,用于查找最大值节点
int compare(int a, int b) {
return a > b ? 1 : (a == b ? 0 : -1);
}
// 查找并返回链表中的最大值节点
Node* findMax(Node* head) {
if (head == NULL) return NULL;
Node* maxNode = head;
while (maxNode->next != NULL) {
if (compare(maxNode->data, maxNode->next->data) < 0) {
maxNode = maxNode->next;
}
}
return maxNode;
}
// 在给定节点后插入新元素
void insertAfterMax(Node** head_ref, int new_data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = new_data;
newNode->next = NULL;
Node* maxNode = findMax(*head_ref);
// 如果链表为空或只有一个元素
if (maxNode == NULL || maxNode->next == NULL) {
newNode->next = *head_ref;
*head_ref = newNode;
} else {
newNode->next = maxNode->next;
maxNode->next = newNode;
}
}
// 打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d -> ", node->data);
node = node->next;
}
printf("NULL\n");
}
int main() {
// 创建示例链表
Node* head = NULL;
Node* second = NULL;
Node* third = NULL;
head = malloc(sizeof(Node));
second = malloc(sizeof(Node));
third = malloc(sizeof(Node));
head->data = 5; head->next = second;
second->data = 10; second->next = third;
third->data = 15; third->next = NULL;
printf("Original list:\n");
printList(head);
// 插入新元素
insertAfterMax(&head, 20);
printf("\nList after inserting at the end of max value:\n");
printList(head);
return 0;
}
```
这个程序首先创建了一个链表,然后在值最大的节点之后插入了新的元素20。注意,这个实现假设输入链表是非空的,并且有多个节点。
阅读全文
相关推荐
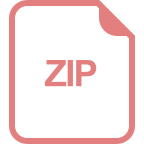
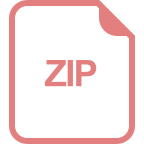







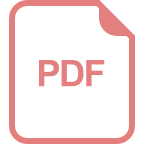
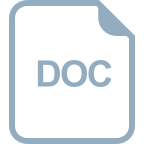






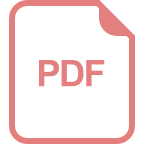