如何用Python编写代码来统计文本中每个单词出现的次数?
时间: 2024-12-16 21:14:54 浏览: 16
在Python中,你可以使用内置的`collections`模块中的`Counter`类来轻松地统计文本中每个单词的出现次数。下面是一个简单的步骤:
1. 首先,你需要将文本分割成单个单词。可以使用`split()`函数,它会根据默认的空格分隔符拆分字符串。
```python
text = "这是一段示例文本,包含一些重复的单词,比如 '示例' 和 '文本'"
words = text.split()
```
2. 然后创建一个`Counter`对象,并传入这个单词列表作为参数。
```python
from collections import Counter
word_counts = Counter(words)
```
3. `word_counts`现在就是一个字典,其中键是单词,值是该单词在文本中出现的次数。
```python
print(word_counts)
```
完整的代码示例:
```python
text = "这是一段示例文本,包含一些重复的单词,比如 '示例' 和 '文本'"
words = text.split()
word_counts = Counter(words)
for word, count in word_counts.items():
print(f"'{word}': {count} 次")
```
相关问题
统计Python文本中每个单词出现的次数。
这个问题可以通过编程实现,你可以使用Python编写一个程序来统计每个单词在文本中出现的次数。以下是一个简单的示例代码:
```python
text = "hello world hello python hello python world"
words = text.split()
word_count = {}
for word in words:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
print(word_count)
```
这个程序将文本中的所有单词存储在一个列表中,然后使用一个字典来记录每个单词出现的次数。最后,打印出每个单词的出现次数。
如何使用Python编写代码来统计mylove.txt文件中每个英文单词的出现次数,并按频率降序打印出前10个单词,同时将这些数据保存到'词频统计.txt'文件中?
你可以使用Python的内置模块`collections`中的`Counter`来统计单词出现次数,再结合`io`模块读取和写入文本文件。这里是一个简单的步骤示例:
```python
import re
from collections import Counter
# 读取mylove.txt文件内容
with open('mylove.txt', 'r', encoding='utf-8') as file:
content = file.read()
# 使用正则表达式提取所有英文单词
words = re.findall(r'\b\w+\b', content.lower()) # 将所有单词转为小写并提取
# 使用Counter计算单词频率
word_counts = Counter(words)
# 按频率排序并获取前10个单词及其频率
top_10_words = word_counts.most_common(10)
# 将结果写入'词频统计.txt'文件
with open('词频统计.txt', 'w', encoding='utf-8') as output_file:
for word, count in top_10_words:
output_file.write(f"{word}: {count}\n")
阅读全文
相关推荐
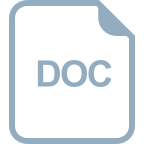
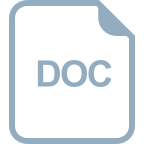
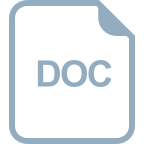



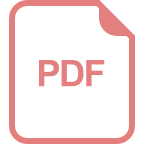
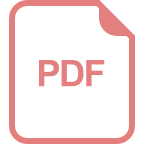









