sha256_final c语言实现
时间: 2023-10-16 07:03:40 浏览: 69
SHA-256是一种加密哈希算法,其用途是将输入的任意长度的数据转换为固定长度的哈希值。在C语言中,我们可以使用以下方式实现SHA-256的最终处理函数:
1. 首先,我们需要定义一些预定义常量和数据结构。这些变量包括SHA-256的初始哈希值,以及一些用于处理消息块的辅助变量。
```c
#include <stdint.h>
// 定义SHA-256初始哈希值
const uint32_t sha256_initial_hash[8] = {
0x6a09e667, 0xbb67ae85, 0x3c6ef372, 0xa54ff53a,
0x510e527f, 0x9b05688c, 0x1f83d9ab, 0x5be0cd19
};
// 定义SHA-256的辅助变量
uint32_t sha256_hash[8];
uint32_t sha256_data[16];
```
2. 实现SHA-256最终处理函数的主体部分。这个函数将在所有的消息块都经过处理后被调用。
```c
#include <string.h>
void sha256_final(unsigned char *hash) {
// 将数据长度转换为比特位
uint64_t bit_length = bit_count * 8;
// 添加一个1和若干个0来填充最后一个消息块
sha256_data[data_index++] = 0x80;
// 检查消息块是否有足够的空间来存储数据长度描述
if (data_index > 14) {
while (data_index < 16) {
sha256_data[data_index++] = 0;
}
sha256_transform();
memset(sha256_data, 0, sizeof(sha256_data));
}
// 最后四个字节存储数据长度(比特位)
sha256_data[14] = bit_length >> 32;
sha256_data[15] = bit_length & 0xffffffff;
// 完成最后一个消息块的处理
sha256_transform();
// 将哈希值复制到输出缓冲区
for (int i = 0; i < 8; ++i) {
hash[i * 4] = sha256_hash[i] >> 24;
hash[i * 4 + 1] = (sha256_hash[i] >> 16) & 0xff;
hash[i * 4 + 2] = (sha256_hash[i] >> 8) & 0xff;
hash[i * 4 + 3] = sha256_hash[i] & 0xff;
}
}
```
以上代码片段演示了SHA-256最终处理函数的C语言实现。在实际中,我们还需要实现其他函数,如数据填充、消息转换等,以完成整个SHA-256算法的实现。
相关推荐
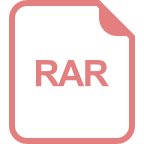














