用C语言建立一棵二叉树,并用递归或非递归的算法分别用先序、中序和后序遍历之。实验要求:(1) 应用先序遍历法建立任意一棵二叉树;并输出(2) 实现二叉树先序、中序、后序遍历的递归算法;(写出代码并运行)
时间: 2024-12-12 16:27:53 浏览: 21
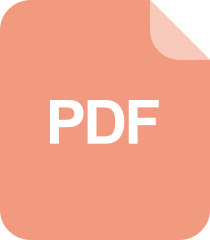
数据结构试验报告用先序中序建立二叉树后序遍历非递归.pdf

首先,让我们来构建一个简单的二叉树节点结构,然后逐步实现先序遍历的创建过程以及递归的前序、中序和后续遍历算法。
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树节点结构
typedef struct Node {
int data;
struct Node* left;
struct Node* right;
} Node;
// 创建新节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->left = newNode->right = NULL;
return newNode;
}
// 先序遍历(根-左-右)
void inorderTraversal(Node* root) {
if (root == NULL) {
return;
}
inorderTraversal(root->left);
printf("%d ", root->data);
inorderTraversal(root->right);
}
// 中序遍历(左-根-右)
void inorderTraversalRecursion(Node* root) {
if (root == NULL) {
return;
}
inorderTraversalRecursion(root->left);
printf("%d ", root->data);
inorderTraversalRecursion(root->right);
}
// 后序遍历(左-右-根)
void postorderTraversal(Node* root) {
if (root == NULL) {
return;
}
postorderTraversal(root->left);
postorderTraversal(root->right);
printf("%d ", root->data);
}
// 用于先序遍历创建二叉树的辅助函数
Node* buildTreePreorder(int arr[], int start, int end) {
if (start > end) {
return NULL;
}
Node* root = createNode(arr[start]);
if (root == NULL) {
return NULL;
}
root->left = buildTreePreorder(arr, start + 1, end);
root->right = buildTreePreorder(arr, start + 2, end);
return root;
}
int main() {
int data[] = {1, 2, 3, 4, 5, 6, 7};
int n = sizeof(data) / sizeof(data[0]);
// 使用先序遍历创建二叉树
Node* root = buildTreePreorder(data, 0, n - 1);
if (root == NULL) {
printf("Error building the tree.\n");
return 1;
}
// 输出先序遍历结果
printf("Preorder traversal: ");
inorderTraversal(root);
printf("\n");
// 非递归中序遍历(使用栈实现)
printf("Inorder traversal (non-recursive): ");
stack<Node*> s;
Node* current = root;
while (current != NULL || !s.isEmpty()) {
while (current != NULL) {
s.push(current);
current = current->left;
}
current = s.pop();
printf("%d ", current->data);
current = current->right;
}
printf("\n");
// 结果输出后,执行递归遍历
printf("Recursive inorder traversal: ");
inorderTraversalRecursion(root);
printf("\n");
printf("Recursive postorder traversal: ");
postorderTraversal(root);
printf("\n");
free(root); // 释放内存
return 0;
}
```
在这个示例中,我们首先定义了一个二叉树节点结构,然后实现了创建节点、先序、中序和后序遍历的函数。`buildTreePreorder`函数使用了递归来根据给定的数组顺序创建二叉树。`main`函数中包含了先序遍历的结果,并展示了如何使用非递归的方式进行中序遍历,以及递归方式进行中序和后序遍历。
阅读全文
相关推荐
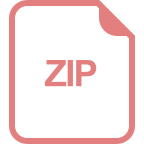
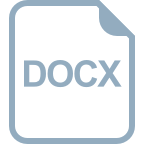















