举一个C++ nv12对齐的代码保存到buffer
时间: 2024-04-12 07:31:02 浏览: 114
下面是一个示例的C++代码,用于将NV12格式的图像数据保存到一个对齐的缓冲区中:
```cpp
#include <iostream>
#include <cstdlib>
#include <cstring>
// 定义对齐宽度
const int ALIGN_WIDTH = 16;
// 对齐函数
int align(int value, int alignment) {
return ((value + alignment - 1) / alignment) * alignment;
}
// 保存NV12数据到对齐的缓冲区
void saveNV12ToBuffer(unsigned char* y, unsigned char* uv, int width, int height, unsigned char* buffer) {
// 计算Y分量的行字节数和对齐后的行字节数
int yRowBytes = width;
int yAlignedRowBytes = align(yRowBytes, ALIGN_WIDTH);
// 计算UV分量的行字节数和对齐后的行字节数
int uvRowBytes = width / 2;
int uvAlignedRowBytes = align(uvRowBytes, ALIGN_WIDTH);
// 计算Y分量数据大小和对齐后的大小
int ySize = yRowBytes * height;
int yAlignedSize = yAlignedRowBytes * height;
// 计算UV分量数据大小和对齐后的大小
int uvSize = uvRowBytes * height / 2;
int uvAlignedSize = uvAlignedRowBytes * height / 2;
// 将Y分量拷贝到对齐的缓冲区中
for (int i = 0; i < height; i++) {
std::memcpy(buffer, y, yRowBytes);
y += yRowBytes;
buffer += yAlignedRowBytes;
}
// 将UV分量拷贝到对齐的缓冲区中
for (int i = 0; i < height / 2; i++) {
std::memcpy(buffer, uv, uvRowBytes);
uv += uvRowBytes;
buffer += uvAlignedRowBytes;
}
}
int main() {
int width = 1920;
int height = 1080;
// 分配原始NV12数据的内存空间
unsigned char* yData = new unsigned char[width * height];
unsigned char* uvData = new unsigned char[width * height / 2];
// 假设已经将NV12数据填充到yData和uvData中
// 计算对齐后的缓冲区大小
int alignedBufferSize = align(width, ALIGN_WIDTH) * height + align(width / 2, ALIGN_WIDTH) * height / 2;
// 分配对齐的缓冲区内存空间
unsigned char* alignedBuffer = new unsigned char[alignedBufferSize];
// 将NV12数据保存到对齐的缓冲区中
saveNV12ToBuffer(yData, uvData, width, height, alignedBuffer);
// 在这里可以使用对齐的缓冲区进行后续处理
// 释放内存空间
delete[] yData;
delete[] uvData;
delete[] alignedBuffer;
return 0;
}
```
在以上示例中,`saveNV12ToBuffer`函数用于将NV12格式的图像数据保存到对齐的缓冲区中。首先,根据图像的宽度、高度和对齐宽度计算Y分量和UV分量的行字节数以及对齐后的行字节数。然后,使用`memcpy`函数将Y分量和UV分量逐行拷贝到对齐的缓冲区中,确保每一行的数据都按照对齐宽度进行对齐。
在`main`函数中,首先分配原始NV12数据的内存空间,并假设已经将NV12数据填充到`yData`和`uvData`中。然后,根据对齐宽度和图像尺寸计算对齐的缓冲区大小,并分配对齐的缓冲区内存空间。最后,调用`saveNV12ToBuffer`函数将NV12数据保存到对齐的缓冲区中。在实际应用中,您可以在对齐的缓冲区上进行后续处理。
请注意,在实际应用中,除了对齐,还需要考虑其他因素,如内存分配和数据访问的正确性。以上示例代码仅仅演示了NV12数据保存到对齐缓冲区的过程,具体的实现还需根据实际需求进行相应的处理。
阅读全文
相关推荐




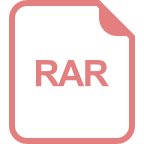


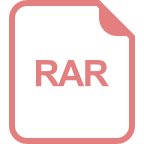

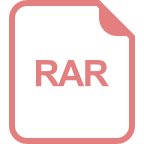

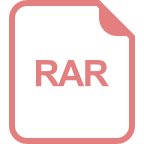



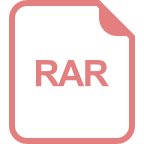
