import numpy as np import pandas as pd data = pd.read_csv("data/预处理.csv", header=None) data = np.array(data) cluster = [] # 按第二个到第十四个标签分类 for i in range(178): if len(cluster) == 0: cluster.append([0]) else: m = 0 for j in range(len(cluster)): n = 0 for x in range(1, 14): if data[i][x] == data[j][x]: n = n + 1 if n == 2: cluster[j].append(i) m = 1 if m == 0: cluster.append([i]) for i in range(len(cluster)): for j in range(len(cluster)): if cluster[i] == cluster[j] and i != j: cluster[j] = 0 # 定义函数来判断两个集合是否相似 def is_similar(set1, set2): for item in set1: if item not in set2: return False return True xiu1 = [] for t in range(178): if data[t][0] == 1: xiu1.append(t) xiu2 = [] for t in range(178): if data[t][0] == 2: xiu2.append(t) xiu3 = [] for t in range(178): if data[t][0] == 3: xiu3.append(t) #修改代码,输出xiu1, xiu2, xiu3在满足13个条件属性下的下近似集合 approx_xiu2 = [t for t in xiu2 if is_similar(data[t][1:14], data[x][1:14]) for x in xiu2] approx_xiu3 = [t for t in xiu3 if is_similar(data[t][1:14], data[x][1:14]) for x in xiu3] print(f"xiu1 在第{col}个条件属性下的下近似集合:", approx_xiu1) print(f"xiu2 在第{col}个条件属性下的下近似集合:", approx_xiu2) print(f"xiu3 在第{col}个条件属性下的下近似集合:", approx_xiu3)
时间: 2024-04-13 14:29:42 浏览: 15
请注意,你的代码缺少对xiu1在每个条件属性下的下近似集合的计算。在你的代码中,你只计算了xiu2和xiu3在每个条件属性下的下近似集合。以下是修改后的代码,包括计算xiu1在每个条件属性下的下近似集合:
```python
import numpy as np
import pandas as pd
data = pd.read_csv("data/预处理.csv", header=None)
data = np.array(data)
cluster = []
# 按第二个到第十四个标签分类
for i in range(178):
if len(cluster) == 0:
cluster.append([0])
else:
m = 0
for j in range(len(cluster)):
n = 0
for x in range(1, 14):
if data[i][x] == data[j][x]:
n = n + 1
if n == 2:
cluster[j].append(i)
m = 1
if m == 0:
cluster.append([i])
for i in range(len(cluster)):
for j in range(len(cluster)):
if cluster[i] == cluster[j] and i != j:
cluster[j] = 0
# 定义函数来判断两个集合是否相似
def is_similar(set1, set2):
for item in set1:
if item not in set2:
return False
return True
xiu1 = []
for t in range(178):
if data[t][0] == 1:
xiu1.append(t)
xiu2 = []
for t in range(178):
if data[t][0] == 2:
xiu2.append(t)
xiu3 = []
for t in range(178):
if data[t][0] == 3:
xiu3.append(t)
# 输出xiu1, xiu2, xiu3在满足13个条件属性下的下近似集合
for col in range(1, 14):
approx_xiu1 = [t for t in xiu1 if is_similar(data[t][1:14], data[x][1:14]) for x in xiu1]
approx_xiu2 = [t for t in xiu2 if is_similar(data[t][1:14], data[x][1:14]) for x in xiu2]
approx_xiu3 = [t for t in xiu3 if is_similar(data[t][1:14], data[x][1:14]) for x in xiu3]
print(f"xiu1 在第{col}个条件属性下的下近似集合:", approx_xiu1)
print(f"xiu2 在第{col}个条件属性下的下近似集合:", approx_xiu2)
print(f"xiu3 在第{col}个条件属性下的下近似集合:", approx_xiu3)
```
现在,代码会输出xiu1、xiu2和xiu3在满足13个条件属性下的下近似集合。希望对你有所帮助!
相关推荐
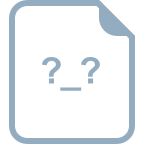
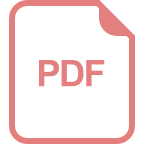
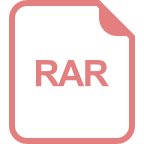














