用c定义一个结构体,包含学生学号、姓名、性别、出生日期,并调用
时间: 2024-09-13 19:06:16 浏览: 46
在C语言中,你可以定义一个结构体(struct)来表示学生的信息,包括学号、姓名、性别和出生日期。下面是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生结构体
typedef struct {
int student_id; // 学号
char name[50]; // 姓名
char gender; // 性别(假设只用大写字母 'M' 或 'F')
time_t birth_date; // 出生日期,使用time_t类型
} Student;
// 功能:创建并初始化一个新的学生实例
Student* create_student(int id, const char* name, char gender_char, time_t birth) {
Student* new_student = (Student*) malloc(sizeof(Student));
if (new_student != NULL) {
new_student->student_id = id;
strncpy(new_student->name, name, sizeof(new_student->name)); // 注意字符串结束符 '\0'
new_student->gender = gender_char == 'M' ? 'M' : 'F';
new_student->birth_date = birth;
return new_student;
}
printf("内存分配失败!\n");
return NULL;
}
int main() {
// 创建并打印示例学生
Student* example_student = create_student(1001, "张三", 'M', time(NULL) - 24 * 60 * 60 * 365); // 假设一年前出生
if (example_student != NULL) {
printf("学号: %d, 姓名: %s, 性别: %c, 出生日期: %ld\n",
example_student->student_id, example_student->name, example_student->gender, (long) example_student->birth_date);
free(example_student); // 记得释放内存
}
return 0;
}
```
阅读全文
相关推荐
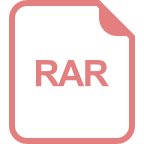
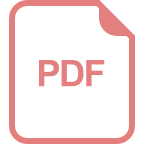
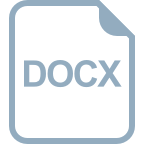















