写一个C++代码解决上面这个问题
时间: 2024-10-06 15:05:21 浏览: 25
为了编写一个C++代码解决这个问题,我们可以采用结构体来存储学生信息,使用一个map来高效地按姓名查找,同时维护一个优先队列(堆)来快速找到分数最低的高分者。这里是一个简化的示例:
```cpp
#include <iostream>
#include <string>
#include <map>
#include <queue>
// 定义学生结构体
struct Student {
std::string name;
int id;
int score;
};
// 比较函数,用于堆中存放
bool compareStudents(Student& a, Student& b) {
return a.score > b.score || (a.score == b.score && a.id < b.id);
}
int main() {
// 创建一个空的map用于存储学生,key为姓名,value为Student对象
std::map<std::string, Student> studentMap;
std::priority_queue<Student, std::vector<Student>, decltype(compareStudents)> minHeap(compareStudents);
// 输入模式
while (true) {
std::string input;
std::cin >> input;
if (input == "Add") {
std::string name, idStr, scoreStr;
std::cin >> name >> idStr >> scoreStr;
int id = std::stoi(idStr), score = std::stoi(scoreStr);
// 添加学生信息
studentMap[name] = {name, id, score};
if (!minHeap.empty() && minHeap.top().score >= score)
minHeap.pop();
minHeap.push({name, id, score});
} else if (input == "Query") {
int targetScore;
std::cin >> targetScore;
std::cout << "Name\tID\tScore\n";
if (minHeap.empty()) {
std::cout << "No students with lower scores found.\n";
} else {
while (!minHeap.empty() && minHeap.top().score <= targetScore) {
auto it = studentMap.find(minHeap.top().name);
if (it != studentMap.end()) {
std::cout << it->first << "\t" << it->second.id << "\t" << it->second.score << "\n";
minHeap.pop();
}
}
}
} else {
break; // 结束输入
}
}
return 0;
}
```
这个程序首先创建了一个学生结构体,然后利用map存储学生信息并用堆保存已知的低分学生。当遇到“Add”指令时,添加新的学生信息并在必要时更新堆;遇到“Query”指令时,从堆中提取符合条件的学生。如果用户输入其他内容,程序结束。
阅读全文
相关推荐
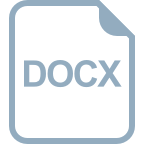
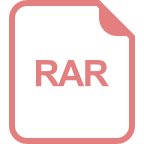
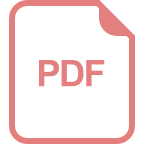















