C# Dictionary检查key重复
时间: 2024-04-13 08:30:36 浏览: 76
在C#中,可以使用Dictionary数据结构来存储键值对,并且可以通过检查键是否存在来避免重复的键。你可以使用Dictionary的ContainsKey方法来检查特定键是否已经存在于字典中。以下是一个示例:
```csharp
Dictionary<string, int> dictionary = new Dictionary<string, int>();
string key = "example";
if (dictionary.ContainsKey(key))
{
// 键已经存在
Console.WriteLine("键已经存在");
}
else
{
// 键不存在
Console.WriteLine("键不存在");
}
```
在上面的示例中,我们创建了一个Dictionary对象并检查了指定的键是否已经存在。如果键存在,我们可以执行相应的操作,否则执行其他操作。
希望这可以帮助你解决问题!如果你有任何其他问题,请随时提问。
相关问题
C# dictionary 通过value查找key值
在 C# 中,Dictionary 类提供了通过值查找键的方法。你可以使用 LINQ 查询语法或循环遍历字典来实现这个功能。以下是两种方法的示例:
1. 使用 LINQ 查询语法:
```csharp
var dictionary = new Dictionary<string, int>
{
{ "apple", 1 },
{ "banana", 2 },
{ "orange", 3 }
};
int targetValue = 2;
string key = dictionary.FirstOrDefault(x => x.Value == targetValue).Key;
if (key != null)
{
Console.WriteLine($"Key with value {targetValue} is: {key}");
}
else
{
Console.WriteLine("Key not found for the given value.");
}
```
2. 使用循环遍历字典:
```csharp
var dictionary = new Dictionary<string, int>
{
{ "apple", 1 },
{ "banana", 2 },
{ "orange", 3 }
};
int targetValue = 2;
string key = null;
foreach (var kvp in dictionary)
{
if (kvp.Value == targetValue)
{
key = kvp.Key;
break;
}
}
if (key != null)
{
Console.WriteLine($"Key with value {targetValue} is: {key}");
}
else
{
Console.WriteLine("Key not found for the given value.");
}
```
这些代码示例将在给定一个目标值时,通过值查找字典中对应的键。请注意,如果字典中存在多个具有相同值的键,这些方法将返回第一个匹配的键。
c# 获取Dictionary某个Key值的数量
在C#中,如果你想要获取`Dictionary<TKey, TValue>`中特定键值的数量,直接使用`Count`属性并传递键作为查询条件是不可行的,因为`Count`方法返回的是整个字典中键值对的数量。然而,你可以通过遍历字典并计数来得到某个特定键对应的具体项数。
例如,假设你想统计键为"myKey"的元素数量,可以这样做:
```csharp
Dictionary<string, int> myDict = new Dictionary<string, int>();
// 假设字典已填充了一些键值对,其中可能包含"myKey"
int countForMyKey = 0;
foreach (KeyValuePair<string, int> pair in myDict)
{
if (pair.Key == "myKey")
{
countForMyKey++;
}
}
int keyValueCount = countForMyKey; // 这就是键为"myKey"的元素数量
```
如果字典很大,这种操作可能会比较耗时。在这种情况下,你可能需要考虑预先排序或者使用其他数据结构来优化查找。
阅读全文
相关推荐


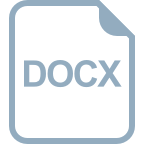
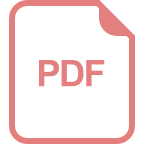
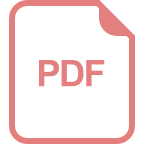
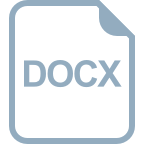
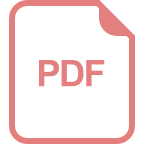
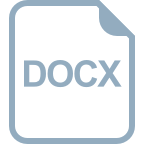
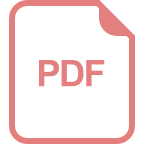
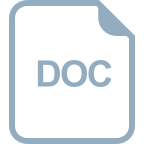



