如何通过C++阻止类的默认拷贝构造和赋值行为?
时间: 2024-11-03 19:19:13 浏览: 60
在C++中,如果你不希望某个类有默认的拷贝构造函数或赋值操作符,你可以声明它们为`private`,然后提供自己的版本(如果需要)。这可以通过以下几种方式实现:
1. **禁用拷贝构造函数**:
```cpp
class MyClass {
public:
// 不提供默认的拷贝构造函数
MyClass(const MyClass&) = delete; // C++11及以上
MyClass(MyClass&&) = default; // 如果你想支持移动构造
private:
MyClass(); // 私有构造函数防止外部实例化
};
```
这里使用了`delete`关键字来表示这个函数是不允许的。
2. **禁用赋值运算符**:
```cpp
class MyClass {
public:
// 不提供默认的赋值操作符
MyClass& operator=(const MyClass&) = delete;
MyClass& operator=(MyClass&&) = delete;
private:
MyClass(); // 私有构造函数防止外部实例化
};
```
3. **提供自己的拷贝/赋值实现**:
如果你想控制拷贝的行为,但又不完全禁止,可以提供一个只执行某些操作的版本。例如,可能你希望深拷贝而不进行浅拷贝。
```cpp
class MyClass {
public:
MyClass(const MyClass& other) : ... { ... } // 自定义拷贝构造函数
MyClass& operator=(const MyClass& other) {
if (this != &other) {
// 执行自定义的深拷贝逻辑
...
}
return *this;
}
private:
MyClass() = default; // 私有构造函数
};
```
记住,这样做可能会限制类的可重用性和兼容性,所以只有在明确的理由下才这么做,比如性能优化或数据安全性考虑。如果没有特殊需求,通常推荐使用默认的行为,除非你知道有更好的替代方案。
阅读全文
相关推荐




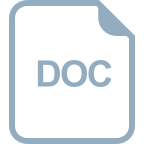













