编写程序,输入待插元素和插入位置,将其插入到单链表中,成功返回1,否则返回0。 输入分三行: 第一行是若干正整数,最后以-1表示结尾(-1不算在序列内,不要处理)。所有数据之间用空格分隔。 第二行数据是插入位置,第三行数据是被插入元素值。用C语言
时间: 2024-10-18 19:24:59 浏览: 20
在C语言中,你可以使用链表数据结构来实现这个功能。首先,你需要定义一个链表节点结构体,包含一个指向下一个节点的指针和一个存储数据的域。然后,你可以按照以下步骤编写程序:
1. 定义链表节点结构:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 初始化链表头结点:
```c
Node* head = NULL;
```
3. 读取输入并插入元素:
```c
int main() {
int n, pos, val;
while (scanf("%d", &n) != EOF && n != -1) { // 遍历输入
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
newNode->data = n;
if (head == NULL) {
head = newNode; // 如果链表为空,将新节点设为头节点
} else {
Node* current = head;
for (int i = 0; i < pos - 1; i++) { // 寻找插入位置
if (current->next != NULL)
current = current->next;
else {
printf("Invalid insertion position.\n"); // 插入位置超出范围,返回错误
return 0;
}
}
newNode->next = current->next; // 将新节点插入适当位置
current->next = newNode; // 更新链表
}
}
// 插入成功,返回1
printf("1\n");
return 1;
}
```
在这个程序中,我们假设插入位置从0开始计数。如果输入的插入位置大于链表的实际长度,我们会提示用户输入无效并返回0。
阅读全文
相关推荐
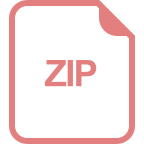
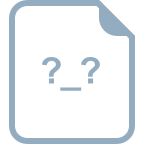
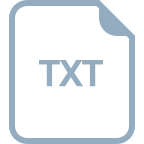
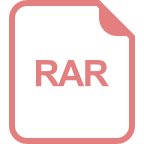
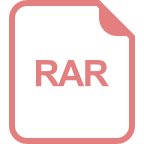
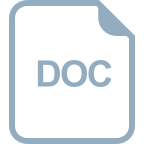
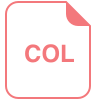
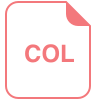
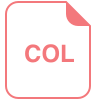
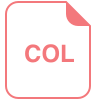







