python输出文件中的单词数量
时间: 2024-09-18 07:13:32 浏览: 67
在Python中,要统计文件中的单词数量,可以使用内置的`open()`函数打开文件,然后逐行读取内容,并使用字符串处理方法来提取单词,最后通过字典或其他数据结构来记录每个单词的出现次数。这里是一个简单的例子:
```python
def count_words_in_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
# 读取文件内容并转换为小写以统一计数
content = file.read().lower()
# 使用空格分隔单词,并将它们添加到集合中去除重复
words = set(content.split())
# 计算单词数量
word_count = len(words)
return word_count
# 示例用法
file_name = "example.txt"
word_count = count_words_in_file(file_name)
print(f"文件 '{file_name}' 中有 {word_count} 个单词.")
```
这个函数会返回文件中不重复单词的数量。注意,这个例子假设文件是以UTF-8编码的,如果文件使用其他编码,需要相应调整。
相关问题
用Python统计文件中单词数量
可以使用Python内置的collections模块中的Counter类来实现单词数量的统计。具体实现步骤如下:
1. 读取文件内容,并将其全部转换为小写字母,以便统计单词数量时不会因为大小写不同而重复计数。
```
with open('filename.txt', 'r') as f:
text = f.read().lower()
```
2. 使用正则表达式去除文本中的标点符号和其他非字母字符,并将文本分割为单词列表。
```
import re
words = re.findall(r'\b\w+\b', text)
```
3. 使用Counter类统计单词数量,并按照出现次数从多到少排序。
```
from collections import Counter
word_counts = Counter(words).most_common()
```
完整代码如下:
```
import re
from collections import Counter
with open('filename.txt', 'r') as f:
text = f.read().lower()
words = re.findall(r'\b\w+\b', text)
word_counts = Counter(words).most_common()
print(word_counts)
```
其中,filename.txt是需要统计单词数量的文件名。最后会输出一个按照单词出现次数从多到少排序的单词列表。
python输出文件中单词的数量,将其中所有标点符号替换为空格,再根据空格对字符串进行切分可以得到元素为单词的列表。数字按单词统计
在Python中,你可以使用`re`模块进行正则表达式操作,先去除标点符号,然后读取文件内容,对每行文本进行处理并统计单词数量。这里是一个简单的步骤:
1. 导入必要的库:
```python
import re
```
2. 定义函数来处理文件内容:
```python
def count_words_in_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
# 使用正则表达式替换标点符号,并转换成小写
cleaned_content = re.sub(r'[^\w\s]', '', content).lower()
# 利用split()函数根据空格分割字符串,得到单词列表
words_list = cleaned_content.split()
# 统计单词频率
word_count = {word: words_list.count(word) for word in words_list}
return word_count
```
3. 调用函数并打印结果:
```python
file_path = "your_file.txt" # 替换为你需要分析的文件路径
word_counts = count_words_in_file(file_path)
for word, count in word_counts.items():
print(f"{word}: {count}")
阅读全文
相关推荐


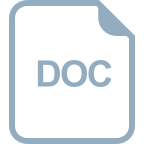













