任务:设计一个生成器函数 count(),每次使用next 函数调用该函数时,数字就会加 1。请编写代码实现一个从 0 开始的计数器。界面输入终止值。
时间: 2024-10-11 17:12:57 浏览: 18
当然可以。你可以创建一个生成器函数`count()`,它会按照用户指定的终止值递增计数。下面是一个简单的Python示例:
```python
def count(start=0, end=None):
current = start
while True:
if end is not None and current >= end:
yield current
break
else:
yield current
current += 1
# 使用示例:
termination_value = int(input("请输入终止值(或直接回车结束计数):"))
counter = count(0, termination_value)
for i in counter:
print(i)
if i == termination_value:
break
```
在这个例子中,`count`函数接收两个参数:开始值`start`,默认为0;终止值`end`。当计数值达到或超过`end`时,函数停止生成下一个数,并通过`break`退出循环。如果没有提供`end`值,计数将无限进行。
如果你想立即结束计数,只需在运行时输入`end`值即可。如果什么都不输入,计数将一直持续到程序中断。
相关问题
编写生成器函数,接受一个字符串,分别统计大写字母,小写字母,数字,其他字符的数量,以元组返回结果
好的,以下是生成器函数的代码:
```python
def count_chars(string):
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
for char in string:
if char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
yield (upper_count, lower_count, digit_count, other_count)
```
这个函数接受一个字符串作为参数,并使用一个循环遍历字符串中的每个字符。然后检查每个字符是否是大写字母、小写字母、数字或其他字符,并相应地增加计数器的值。
最后,函数使用 `yield` 语句将一个元组返回,其中包含每个计数器的值。请注意,由于这是一个生成器函数,所以我们可以使用 `next()` 函数来获取它的结果,如下所示:
```python
string = "Hello World! 123"
result = count_chars(string)
print(next(result)) # 输出 (2, 8, 3, 2)
```
这里的 `next()` 函数调用将执行 `count_chars()` 函数,并返回它生成的第一个元组。在这个例子中,结果是 `(2, 8, 3, 2)`,表示字符串中有 2 个大写字母,8 个小写字母,3 个数字和 2 个其他字符。
编写生成器函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以元组的形式返回结果
好的,下面是生成器函数的代码实现:
```python
def count_chars(s):
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
for c in s:
if c.isupper():
upper_count += 1
elif c.islower():
lower_count += 1
elif c.isdigit():
digit_count += 1
else:
other_count += 1
yield (upper_count, lower_count, digit_count, other_count)
```
这个函数接收一个字符串 `s`,然后用一个循环遍历字符串中的每个字符。根据字符的类型分别累加大写字母、小写字母、数字和其他字符的个数。最后用 `yield` 语句返回一个元组,包含各种字符的个数。注意这里使用了生成器函数,所以当你调用这个函数时,实际上只是得到一个生成器对象,需要使用 `next()` 函数来获取生成器的下一个值,或者使用 `for` 循环来迭代生成器对象。
阅读全文
相关推荐
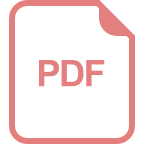
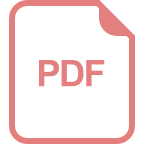
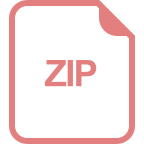













