1、写一个函数,实现链表的排序(使链表中元素的从无序到有序,要求从小到大); 我的作业
时间: 2024-03-04 12:47:49 浏览: 120
好的,我可以帮你解答这个问题。下面是一个基于归并排序的链表排序的示例代码。
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def merge(l1: ListNode, l2: ListNode) -> ListNode:
dummy = ListNode(0)
cur = dummy
while l1 and l2:
if l1.val < l2.val:
cur.next = l1
l1 = l1.next
else:
cur.next = l2
l2 = l2.next
cur = cur.next
cur.next = l1 if l1 else l2
return dummy.next
def sortList(head: ListNode) -> ListNode:
if not head or not head.next:
return head
slow, fast = head, head.next
while fast and fast.next:
slow = slow.next
fast = fast.next.next
mid = slow.next
slow.next = None
left = sortList(head)
right = sortList(mid)
return merge(left, right)
```
其中,merge() 函数是用来合并两个已排序链表的函数,sortList() 函数则是使用归并排序的方式对链表进行排序。时间复杂度为 O(nlogn)。
使用示例:
```python
# 构造链表
head = ListNode(4)
node1 = ListNode(2)
node2 = ListNode(1)
node3 = ListNode(3)
head.next = node1
node1.next = node2
node2.next = node3
# 对链表进行排序
head_sorted = sortList(head)
# 打印排序后的链表
cur = head_sorted
while cur:
print(cur.val, end=' ')
cur = cur.next
# 输出:1 2 3 4
```
希望能够帮助你完成作业。
阅读全文
相关推荐
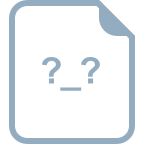




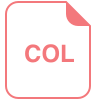
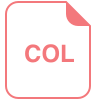
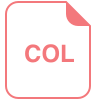
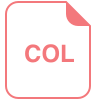
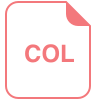
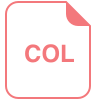
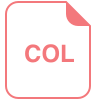
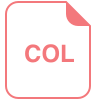
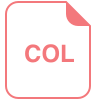
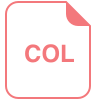
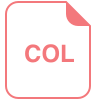