C语言互斥锁一般的函数有什么
时间: 2024-11-09 18:27:11 浏览: 12
在C语言中,互斥锁(Mutex)通常用于控制并发访问共享资源,保证在同一时间只有一个线程能访问它。标准库中并没有直接提供互斥锁的函数,但是你可以通过头文件`pthread.h`来使用POSIX线程(Pthreads)提供的功能来实现互斥锁。以下是几个常用的函数:
1. `pthread_mutex_init()`:初始化一个新的互斥锁。
```c
pthread_mutex_t mutex; // 创建一个互斥锁变量
int result = pthread_mutex_init(&mutex, NULL); // 初始化互斥锁
```
2. `pthread_mutex_lock()`:获取互斥锁,如果已被其他线程锁定,则阻塞。
```c
if (pthread_mutex_lock(&mutex) != 0) {
// 错误处理
}
```
3. `pthread_mutex_unlock()`:释放已经获得的互斥锁,允许其他线程获取。
```c
pthread_mutex_unlock(&mutex); // 当完成对共享资源的操作后解锁
```
4. `pthread_mutex_destroy()`:销毁已初始化的互斥锁。
```c
pthread_mutex_destroy(&mutex); // 线程结束前需要删除互斥锁
```
相关问题
linux中c语言互斥锁
Linux中的C语言互斥锁是一种同步机制,用于保护共享资源不受并发访问的影响。它可以确保在任何时刻只有一个线程可以访问被保护的共享资源,从而避免了数据竞争和死锁等问题。
互斥锁有两种状态:锁定和非锁定。当一个线程请求锁定一个互斥锁时,如果互斥锁当前处于非锁定状态,那么该线程将获得锁并继续执行;如果互斥锁当前处于锁定状态,那么该线程将被阻塞,直到该互斥锁被解锁为止。
在Linux中,使用pthread_mutex_init()函数初始化互斥锁,使用pthread_mutex_lock()函数锁定互斥锁,使用pthread_mutex_unlock()函数解锁互斥锁。
C语言 互斥锁用法举例
以下是一个使用互斥锁的简单示例:
#include <stdio.h>
#include <pthread.h>
int count = 0;
pthread_mutex_t mutex;
void *increment(void *arg) {
int i;
for (i = 0; i < 1000000; i++) {
pthread_mutex_lock(&mutex);
count++;
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
pthread_t thread1, thread2;
pthread_mutex_init(&mutex, NULL);
pthread_create(&thread1, NULL, increment, NULL);
pthread_create(&thread2, NULL, increment, NULL);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
pthread_mutex_destroy(&mutex);
printf("Count: %d\n", count);
return 0;
}
在此示例中,我们创建了一个名为“count”的全局变量和一个互斥锁变量“mutex”。我们使用两个线程并行地增加计数器“count”的值。
在每个线程的主体中,我们使用pthread_mutex_lock函数获取互斥锁,然后增加计数器的值,最后使用pthread_mutex_unlock函数释放互斥锁。
通过使用互斥锁,我们确保每个线程不会同时访问共享资源(计数器),从而避免了竞争条件和数据不一致的问题。最后,我们输出计数器的值以检查它是否正确增加。
阅读全文
相关推荐
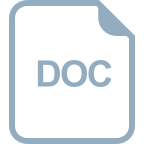
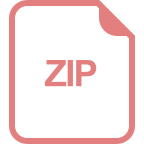
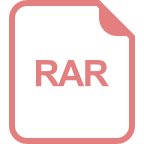
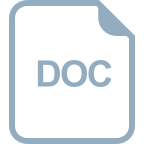
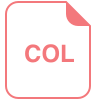




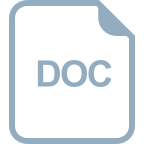
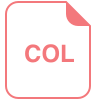
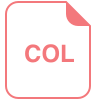




