C# 与PLC通信 代码
时间: 2023-10-23 18:12:42 浏览: 92
以下是一个基于OPC UA协议的C#与PLC通信的代码示例:
```csharp
using Opc.Ua;
using Opc.Ua.Client;
using System;
class Program
{
static void Main(string[] args)
{
// 创建一个OPC UA客户端实例
var client = new OpcClient();
// 连接到PLC
client.Connect("opc.tcp://localhost:4840");
// 读取PLC中的变量值
var node = client.ReadNode("ns=2;s=MyVariable");
Console.WriteLine("Variable value: " + node.Value);
// 写入变量值到PLC
client.WriteNode("ns=2;s=MyVariable", "new value");
// 断开与PLC的连接
client.Disconnect();
}
}
public class OpcClient
{
private Session session;
public void Connect(string endpointUrl)
{
// 创建一个OPC UA会话实例
var endpoint = new ConfiguredEndpoint(null, new EndpointDescription(endpointUrl));
var session = Session.Create(
new SessionConfiguration(),
new ConfiguredEndpointCollection { endpoint },
false,
false,
null,
null);
// 连接到PLC
session.Open();
this.session = session;
}
public void Disconnect()
{
// 断开与PLC的连接
session?.Close();
session?.Dispose();
session = null;
}
public DataValue ReadNode(string nodeId)
{
// 读取PLC中的变量值
var node = new NodeId(nodeId);
var value = session.ReadValue(node);
return value;
}
public void WriteNode(string nodeId, object value)
{
// 写入变量值到PLC
var node = new NodeId(nodeId);
var dataValue = new DataValue(new Variant(value));
session.WriteValue(node, dataValue);
}
}
```
需要注意的是,以上代码仅为示例,实际使用时需要根据PLC的具体配置进行相应的修改。
阅读全文
相关推荐
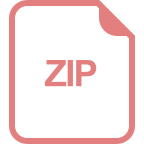
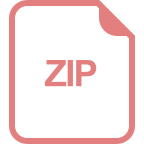
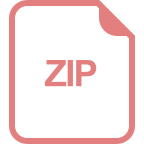















