能详细说一下关于landmark labels用于姿态估计的用法吗,并提供示例代码
时间: 2024-03-21 09:39:28 浏览: 147
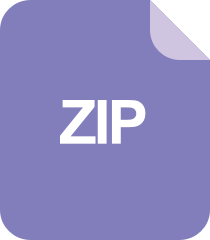
基于Mediapipe的人体姿态识别与匹配计算python源码+项目使用说明+超详细注释.zip

当使用DeepFashion数据集中的Landmark Labels标注进行姿态估计时,可以使用以下步骤:
1. 首先,我们需要读取图像和标注文件,并将标注数据转换为关键点坐标的列表。
```python
import cv2
import numpy as np
# 读取图像和标注文件
img_path = "image.jpg"
anno_path = "annotation.txt"
img = cv2.imread(img_path)
# 从标注文件中提取关键点坐标
with open(anno_path, "r") as f:
data = f.read().splitlines()
keypoints = []
for i in range(len(data)):
if i == 0:
continue
x, y = data[i].split(" ")
keypoints.append((int(x), int(y)))
```
2. 接着,我们需要构建人体模型。在这里,我们使用OpenCV提供的cv2.solvePnP函数来实现。
```python
# 定义人体模型中的关键点
model_points = np.array([
(0, 0, 0), # 鼻子
(0, -330, 0), # 下巴
(-225, 170, 0), # 左眼角
(225, 170, 0), # 右眼角
(-150, -150, 0), # 左嘴角
(150, -150, 0) # 右嘴角
])
# 定义相机矩阵和畸变系数
camera_matrix = np.array([
[1.0, 0, img.shape[1]/2],
[0, 1.0, img.shape[0]/2],
[0, 0, 1.0]
])
dist_coeffs = np.zeros((4, 1))
# 使用cv2.solvePnP函数计算旋转和平移向量
success, rotation_vector, translation_vector = cv2.solvePnP(
model_points, np.array(keypoints), camera_matrix, dist_coeffs)
# 将旋转向量转换为旋转矩阵
rotation_matrix, _ = cv2.Rodrigues(rotation_vector)
```
3. 最后,我们可以使用旋转矩阵和平移向量来对图像进行姿态估计。
```python
# 构建投影矩阵
proj_matrix = np.hstack((rotation_matrix, translation_vector))
# 定义立方体的8个顶点
box = np.array([
[-50, -50, -50],
[-50, -50, 50],
[-50, 50, -50],
[-50, 50, 50],
[50, -50, -50],
[50, -50, 50],
[50, 50, -50],
[50, 50, 50]
])
# 将立方体投影到图像上
box_2d = cv2.projectPoints(box, rotation_vector, translation_vector, camera_matrix, dist_coeffs)[0]
# 绘制立方体
for i in range(4):
cv2.line(img, tuple(box_2d[i][0]), tuple(box_2d[i+4][0]), (0, 255, 0), 2)
cv2.line(img, tuple(box_2d[i][0]), tuple(box_2d[(i+1)%4][0]), (0, 255, 0), 2)
cv2.line(img, tuple(box_2d[i+4][0]), tuple(box_2d[(i+1)%4+4][0]), (0, 255, 0), 2)
# 显示图像
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们首先使用cv2.solvePnP函数计算出人体的旋转和平移向量,然后将旋转向量转换为旋转矩阵。接着,我们使用旋转矩阵和平移向量来构建投影矩阵,并将立方体投影到图像上。最后,我们使用cv2.line函数将立方体绘制到图像上,并显示图像。
需要注意的是,上面的代码只是一个简单的示例,实际上姿态估计的算法可能会更加复杂,需要根据具体情况进行调整。
阅读全文
相关推荐
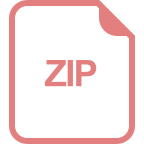

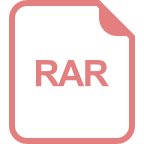
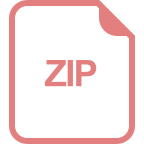
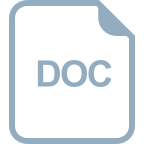
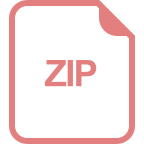










