net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")如何获取
时间: 2024-01-12 22:02:17 浏览: 380
YOLOv3模型的权重文件和配置文件?
要获取YOLOv3模型的权重文件和配置文件,首先需要在Darknet官网下载它们。权重文件用于指定模型的权重和偏差,可以从链接 https://pjreddie.com/media/files/yolov3.weights 下载。配置文件则用于指定模型的层结构和超参数,可以从链接 https://github.com/pjreddie/darknet/blob/master/cfg/yolov3.cfg 下载。下载完成后,将它们放在你的项目文件夹中,并使用cv2.dnn.readNet函数加载它们来构建YOLOv3模型。
相关问题
import cv2 from gui_buttons import Buttons # Initialize Buttons button = Buttons() button.add_button("cup", 20,100) button.add_button("phone", 20, 20) button.add_button("book", 20, 180) colors = button.colors # Opencv DNN net = cv2.dnn.readNet("dnn_model/yolov4-tiny.weights", "dnn_model/yolov4-tiny.cfg") model = cv2.dnn_DetectionModel(net) model.setInputParams(size=(320, 320), scale=1/255) # Load class lists classes = [] with open("dnn_model/classes.txt", "r") as file_object: for class_name in file_object.readlines(): class_name = class_name.strip() classes.append(class_name) print("Objects list") print(classes) # Initialize camera cap = cv2.VideoCapture(0, cv2.CAP_DSHOW)#( 'G:\course_320\视频素材参考\CF.mp4') # 2, cv2.CAP_DSHOW cap.set(cv2.CAP_PROP_FRAME_WIDTH, 1200) cap.set(cv2.CAP_PROP_FRAME_HEIGHT,650) # FULL HD 1920 x 1080 def click_button(event, x, y, flags, params): global button_person if event == cv2.EVENT_LBUTTONDOWN: button.button_click(x, y) # Create window cv2.namedWindow("Frame") cv2.setMouseCallback("Frame", click_button) while True: # Get frames ret, frame = cap.read() # Get active buttons list active_buttons = button.active_buttons_list() #print("Active buttons", active_buttons) # Object Detection (class_ids, scores, bboxes) = model.detect(frame, confThreshold=0.3, nmsThreshold=0.4) for class_id, score, bbox in zip(class_ids, scores, bboxes): (x, y, w, h) = bbox class_name = classes[class_id] color = colors[class_id] if class_name in active_buttons: cv2.putText(frame, class_name, (x, y - 10), cv2.FONT_HERSHEY_PLAIN, 2, color, 2) cv2.rectangle(frame, (x, y), (x + w, y + h), color, 5) # Display buttons button.display_buttons(frame) cv2.imshow("Frame", frame) key = cv2.waitKey(1) if key == 30: break cap.release() cv2.destroyAllWindows()能解释这段代码各部分的功能吗
这段代码实现了一个物体检测的应用,并且加入了交互式的按钮功能。下面是各部分的具体功能:
1. 导入cv2库和自定义的Buttons类,以及其他需要的模块。
2. 初始化按钮对象,添加三个按钮,设置其位置。
3. 获取按钮的颜色。
4. 初始化OpenCV的深度神经网络模型,并加载相应的权重文件和配置文件。
5. 加载类别列表。
6. 初始化摄像头,并设置其分辨率。
7. 定义点击按钮的回调函数,用于处理鼠标事件。
8. 创建窗口,并设置鼠标回调函数。
9. 进入循环,读取视频帧。
10. 获取当前激活的按钮列表。
11. 对视频帧进行物体检测,获取检测结果。
12. 遍历检测结果,如果检测到的物体在激活的按钮列表中,则在视频帧上绘制检测框和类别名称。
13. 显示按钮。
14. 显示视频帧。
15. 等待用户按下“ESC”键退出程序。
16. 释放摄像头资源,关闭窗口。
优化#加载YOLO模型和不同标签 net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg") classes = [] with open("coco.names", "r") as f: classes = [line.strip() for line in f.readlines()]使用yolo格式的数据集
为了优化加载YOLO模型和不同标签,你可以使用OpenCV的dnn模块,通过使用dnn模块,你可以加载模型和标签文件,并使用它们对图像进行目标检测。这里是一个例子:
```
import cv2
# 加载模型和标签
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 配置模型参数
net.setPreferableBackend(cv2.dnn.DNN_BACKEND_OPENCV)
net.setPreferableTarget(cv2.dnn.DNN_TARGET_CPU)
# 加载图像
image = cv2.imread("image.jpg")
# 创建输入blob
blob = cv2.dnn.blobFromImage(image, 1/255, (416, 416), swapRB=True, crop=False)
# 设置输入blob
net.setInput(blob)
# 运行前向传播
outs = net.forward(net.getUnconnectedOutLayersNames())
# 解析输出结果
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * image.shape[1])
center_y = int(detection[1] * image.shape[0])
width = int(detection[2] * image.shape[1])
height = int(detection[3] * image.shape[0])
left = int(center_x - width / 2)
top = int(center_y - height / 2)
class_ids.append(class_id)
confidences.append(float(confidence))
boxes.append([left, top, width, height])
# 非极大值抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制检测结果
for i in indices:
i = i[0]
box = boxes[i]
left = box[0]
top = box[1]
width = box[2]
height = box[3]
cv2.rectangle(image, (left, top), (left+width, top+height), (0, 255, 0), 2)
cv2.putText(image, classes[class_ids[i]], (left+5, top+20), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Detection result", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们首先使用 `cv2.dnn.readNet()` 函数加载YOLO模型和标签文件。然后,我们使用 `cv2.dnn.blobFromImage()` 函数创建输入blob,并将其设置为模型的输入。接下来,我们运行前向传播并解析输出结果。最后,我们使用 `cv2.dnn.NMSBoxes()` 函数进行非极大值抑制,并绘制检测结果。
你可以根据自己的需求修改输入图像和模型参数等。
阅读全文
相关推荐
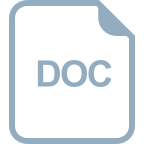
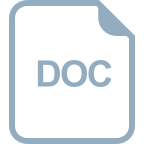
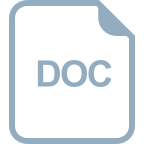
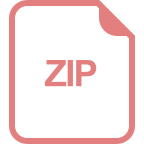
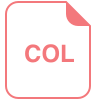
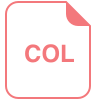
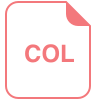
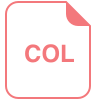
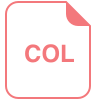







