多文件C++21点游戏
时间: 2023-08-25 17:05:49 浏览: 51
好的,这里是一个用多文件编写的C++ 21点游戏示例程序。
首先,我们将把游戏逻辑和UI分别放在两个不同的文件中。在 `game.cpp` 文件中,我们将定义 `Game` 类,该类将处理游戏逻辑。在 `ui.cpp` 文件中,我们将定义 `UI` 类,该类将处理用户界面。
### game.cpp
```c++
#include <iostream>
#include <vector>
#include <ctime>
#include <cstdlib>
#include "game.h"
using namespace std;
Game::Game() {
srand(time(NULL));
deck = vector<int>(52, 0);
shuffleDeck();
playerCards = vector<int>();
dealerCards = vector<int>();
}
void Game::shuffleDeck() {
for (int i = 0; i < 52; i++) {
deck[i] = i;
}
for (int i = 51; i > 0; i--) {
int j = rand() % (i + 1);
swap(deck[i], deck[j]);
}
}
int Game::getCardValue(int card) {
int value = (card % 13) + 1;
if (value > 10) {
value = 10;
}
return value;
}
int Game::getHandValue(vector<int>& cards) {
int value = 0;
int numAces = 0;
for (int i = 0; i < cards.size(); i++) {
int cardValue = getCardValue(cards[i]);
if (cardValue == 1) {
numAces++;
}
value += cardValue;
}
while (numAces > 0 && value + 10 <= 21) {
value += 10;
numAces--;
}
return value;
}
int Game::dealCard() {
int card = deck.back();
deck.pop_back();
return card;
}
void Game::dealInitialCards() {
playerCards.push_back(dealCard());
dealerCards.push_back(dealCard());
playerCards.push_back(dealCard());
dealerCards.push_back(dealCard());
}
bool Game::isBlackjack(vector<int>& cards) {
return cards.size() == 2 && getHandValue(cards) == 21;
}
bool Game::isBust(vector<int>& cards) {
return getHandValue(cards) > 21;
}
bool Game::isDealerDone() {
return getHandValue(dealerCards) >= 17;
}
void Game::playerHit() {
playerCards.push_back(dealCard());
}
void Game::dealerHit() {
dealerCards.push_back(dealCard());
}
int Game::getPlayerScore() {
return getHandValue(playerCards);
}
int Game::getDealerScore() {
return getHandValue(dealerCards);
}
int Game::getPlayerCardCount() {
return playerCards.size();
}
int Game::getDealerCardCount() {
return dealerCards.size();
}
bool Game::isGameOver() {
return isBust(playerCards) || isBlackjack(playerCards) || !isDealerDone();
}
int Game::getGameResult() {
if (isBust(playerCards)) {
return -1;
} else if (isBlackjack(playerCards) && isBlackjack(dealerCards)) {
return 0;
} else if (isBlackjack(playerCards)) {
return 1;
} else if (isBlackjack(dealerCards)) {
return -1;
} else if (isBust(dealerCards)) {
return 1;
} else if (getHandValue(playerCards) > getHandValue(dealerCards)) {
return 1;
} else if (getHandValue(playerCards) < getHandValue(dealerCards)) {
return -1;
} else {
return 0;
}
}
```
在 `game.h` 文件中,我们将定义 `Game` 类的接口。
### game.h
```c++
#include <vector>
class Game {
public:
Game();
void shuffleDeck();
int getCardValue(int card);
int getHandValue(std::vector<int>& cards);
int dealCard();
void dealInitialCards();
bool isBlackjack(std::vector<int>& cards);
bool isBust(std::vector<int>& cards);
bool isDealerDone();
void playerHit();
void dealerHit();
int getPlayerScore();
int getDealerScore();
int getPlayerCardCount();
int getDealerCardCount();
bool isGameOver();
int getGameResult();
private:
std::vector<int> deck;
std::vector<int> playerCards;
std::vector<int> dealerCards;
};
```
现在,我们将编写处理用户界面的 `UI` 类。在 `ui.cpp` 文件中,我们将定义 `UI` 类,该类将处理用户界面。
### ui.cpp
```c++
#include <iostream>
#include "game.h"
#include "ui.h"
using namespace std;
UI::UI() {
game = Game();
}
void UI::start() {
cout << "Welcome to 21!" << endl;
while (true) {
playRound();
if (!playAgain()) {
break;
}
}
}
void UI::playRound() {
game.shuffleDeck();
game.dealInitialCards();
cout << "Player's cards: ";
printCards(game.playerCards);
cout << "Dealer's cards: ";
printDealerCards(game.dealerCards);
while (true) {
if (game.isGameOver()) {
break;
}
if (getPlayerAction() == 'h') {
game.playerHit();
cout << "Player's cards: ";
printCards(game.playerCards);
} else {
break;
}
}
while (!game.isGameOver()) {
game.dealerHit();
cout << "Dealer's cards: ";
printDealerCards(game.dealerCards);
}
int result = game.getGameResult();
if (result == 1) {
cout << "You win!" << endl;
} else if (result == -1) {
cout << "You lose!" << endl;
} else {
cout << "Push!" << endl;
}
}
char UI::getPlayerAction() {
char action;
while (true) {
cout << "Do you want to (h)it or (s)tand? ";
cin >> action;
if (action == 'h' || action == 's') {
break;
}
}
return action;
}
bool UI::playAgain() {
char response;
while (true) {
cout << "Do you want to play again? (y/n) ";
cin >> response;
if (response == 'y' || response == 'n') {
break;
}
}
return response == 'y';
}
void UI::printCards(vector<int>& cards) {
for (int i = 0; i < cards.size(); i++) {
cout << getCardString(cards[i]) << " ";
}
cout << "(" << game.getHandValue(cards) << ")" << endl;
}
void UI::printDealerCards(vector<int>& cards) {
cout << getCardString(cards[0]) << " X";
for (int i = 2; i < cards.size(); i++) {
cout << " " << getCardString(cards[i]);
}
cout << endl;
}
string UI::getCardString(int card) {
static string suits[] = {"C", "D", "H", "S"};
static string ranks[] = {"A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"};
return ranks[card % 13] + suits[card / 13];
}
```
在 `ui.h` 文件中,我们将定义 `UI` 类的接口。
### ui.h
```c++
#include <vector>
#include <string>
#include "game.h"
class UI {
public:
UI();
void start();
private:
Game game;
void playRound();
char getPlayerAction();
bool playAgain();
void printCards(std::vector<int>& cards);
void printDealerCards(std::vector<int>& cards);
std::string getCardString(int card);
};
```
现在,我们需要创建一个入口点,该入口点将创建 `UI` 对象并调用 `start` 方法。
### main.cpp
```c++
#include "ui.h"
int main() {
UI ui;
ui.start();
return 0;
}
```
现在我们已经完成了一个用多个文件编写的21点游戏示例程序。
相关推荐
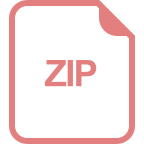
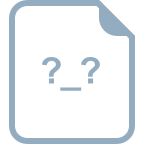















