import threading import cv2 import tkinter as tk from tkinter import * from PIL import Image, ImageTk#图像控件 from Background.Thread_Demo import Thread_data from Data_demo import data_demo class Camera(): def __init__(self, top): self.top = top self.h = int(data_demo.window_height // 1.5) self.w = int(data_demo.window_width // 1.74) self.canvas2 = Canvas(self.top, bg='LightSkyBlue', width=self.w, height=self.h, highlightthickness=2, highlightbackground='Black') self.canvas2.place(relx=0.0, rely=0.032) self.label = tk.Label(self.canvas2, text='摄像头显示区!', font=("黑体", 25), width=15, height=1) self.label.place(relx=0.32, rely=0.50, anchor='nw') def Firing_run(self, button_id): self.bool = True self.label.destroy() self.cap = cv2.VideoCapture(0) t = threading.Thread(target=self.windows_display) t.start() button_id.config(text='关闭摄像头', command=lambda: self.Cease_stop(button_id)) def Cease_stop(self, button_id): self.bool = False button_id.config(text='打开摄像头', command=lambda: self.Firing_run(button_id)) def windows_display(self): while self.bool: ref, frame = self.cap.read() cv2.waitKey(1) if ref: image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) image1 = Image.fromarray(image) image2 = image1.resize((self.w, self.h), Image.ANTIALIAS) self.photo = ImageTk.PhotoImage(image=image2) self.canvas2.create_image(0, 0, image=self.photo, anchor=tk.NW) # self.canvas2.update() self.canvas2.after(15)中t = threading.Thread(target=self.windows_display)调用摄像头会闪烁,但是t = threading.Thread(target=self.windows_display())却不会
时间: 2024-02-14 17:18:09 浏览: 143
原因是当您使用`t = threading.Thread(target=self.windows_display)`时,它会将`self.windows_display()`作为一个参数,而不是一个可调用的函数。因此,线程将立即运行`self.windows_display()`函数,并将其返回值(None)传递给`t`。这意味着您的线程实际上不会运行`self.windows_display()`函数,而是只运行了一次,并立即停止。
相反,当您使用`t = threading.Thread(target=self.windows_display)`时,它会将`self.windows_display`作为一个可调用的函数传递给`t`,因此线程将运行`self.windows_display`函数,并在后台持续运行。
因此,您应该修改线程创建代码如下:
```
t = threading.Thread(target=self.windows_display)
```
而不是:
```
t = threading.Thread(target=self.windows_display())
```
相关问题
import threading import cv2 import tkinter as tk from tkinter import * from PIL import Image, ImageTk#图像控件 from Background.Thread_Demo import Thread_data from Data_demo import data_demo class Two_Layout(): def __init__(self, top): self.top = top self.canvas2 = Canvas(self.top, bg='LightSkyBlue', width=1100, height=610, highlightthickness=2, highlightbackground='Black') self.canvas2.place(x=0, y=28) data_demo.display_id = self.canvas2 Thread_data(self.Thread_()) def Thread_(self): self.cap = cv2.VideoCapture(0) t = threading.Thread(target=self.windows_display()) t.start() def windows_display(self): ref, frame = self.cap.read() data_demo.display_id.delete('all') label = tk.Label(data_demo.display_id, text='未接入摄像头!', font=("黑体", 25), width=15, height=1) if ref is False: label.place(x=400, y=300, anchor='nw') self.cap = cv2.VideoCapture(0) else: for widget in data_demo.display_id.winfo_children(): widget.destroy() image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) image1 = Image.fromarray(image) image2 = image1.resize((1100, 645), Image.ANTIALIAS) self.photo = ImageTk.PhotoImage(image=image2) data_demo.display_id.create_image(0, 0, image=self.photo, anchor=tk.NW) data_demo.display_id.after(15, self.windows_display)为什么还是与filedialog.askopenfilename()起了冲突
你的代码中没有引入 `filedialog`,所以很难确定具体原因。但是,在你的代码中,没有直接调用 `filedialog.askopenfilename()`,所以很可能是其他部分的代码和 `filedialog` 产生了冲突。请检查你的代码并确定是否有其他部分调用了 `filedialog.askopenfilename()`。另外,检查你的代码是否正确导入了 `filedialog`。如果你仍然无法解决问题,请提供更多的细节和代码,以便我能够更好地帮助你。
import cv2 import tkinter as tk from tkinter import * from PIL import Image, ImageTk#图像控件 from Background.Thread_Demo import Thread_data from Data_demo import data_demo class Camera(): def __init__(self, top): self.top = top self.h = int(data_demo.window_height // 1.5) self.w = int(data_demo.window_width // 1.74) self.canvas2 = Canvas(self.top, bg='LightSkyBlue', width=self.w, height=self.h, highlightthickness=2, highlightbackground='Black') self.canvas2.place(relx=0.0, rely=0.032) self.label = tk.Label(self.canvas2, text='摄像头显示区!', font=("黑体", 25), width=15, height=1) self.label.place(relx=0.38, rely=0.45, anchor='nw') # 启动摄像头按钮控件 def Firing_Camera(self): self.label.config(text="等待连接....") self.Firing_Camera1() def Firing_Camera1(self): self.cap = cv2.VideoCapture(0) ret, frame = self.cap.read() if ret: self.running = True self.thread = threading.Thread(target=self.update, args=()) self.thread.start() else: messagebox.showinfo('提示', '请检查摄像头是否接入或状态是否正常') def update(self): while self.running: ret, frame = self.cap.read() if ret: # process the frame here # ... # convert the frame to an RGB image image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) image = Image.fromarray(image) # update the tkinter label for widget in self.canvas2.winfo_children(): widget.destroy() image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) image1 = Image.fromarray(image) image2 = image1.resize((self.w, self.h), Image.ANTIALIAS) self.photo = ImageTk.PhotoImage(image=image2) self.canvas2.create_image(0, 0, image=self.photo, anchor=tk.NW) # wait for a fixed amount of time before capturing the next frame self.canvas2.after(15, self.update)为什么摄像头不动
可能是以下原因导致摄像头不动:
1. 摄像头没有接好或者连接错误,需要检查摄像头连接是否正确。可以尝试更换USB接口或者更换摄像头试试。
2. 摄像头的驱动程序没有安装或者不兼容,需要安装或更新摄像头驱动程序。
3. 摄像头被其他程序占用,需要关闭其他占用摄像头的程序,或者重启电脑后再尝试启动摄像头。
4. 摄像头硬件故障,需要更换摄像头或者修理摄像头。
阅读全文
相关推荐
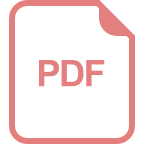
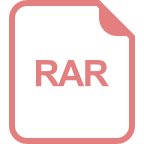
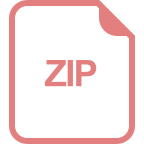




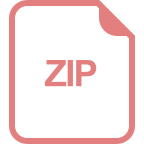
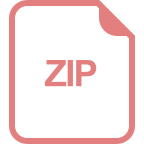
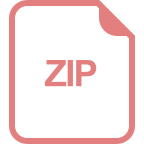
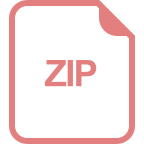
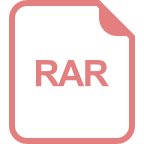
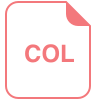



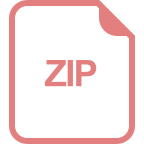