代替if else的方法
时间: 2023-12-07 10:37:38 浏览: 62
以下是几种代替if else语句的方法:
1.使用字典映射:
```python
def dispatch_if(operator, x, y):
if operator == 'add':
return x + y
elif operator == 'sub':
return x - y
elif operator == 'mul':
return x * y
elif operator == 'div':
return x / y
else:
return None
def dispatch_dict(operator, x, y):
return {
'add': lambda: x + y,
'sub': lambda: x - y,
'mul': lambda: x * y,
'div': lambda: x / y,
}.get(operator, lambda: None)()
print(dispatch_if('add', 2, 8)) # 输出:10
print(dispatch_dict('add', 2, 8)) # 输出:10
```
2.使用多态:
```python
class Operator:
def operate(self, x, y):
pass
class AddOperator(Operator):
def operate(self, x, y):
return x + y
class SubOperator(Operator):
def operate(self, x, y):
return x - y
class MulOperator(Operator):
def operate(self, x, y):
return x * y
class DivOperator(Operator):
def operate(self, x, y):
return x / y
def dispatch_polymorphism(operator, x, y):
return operator.operate(x, y)
print(dispatch_polymorphism(AddOperator(), 2, 8)) # 输出:10
```
3.使用函数映射:
```python
def add(x, y):
return x + y
def sub(x, y):
return x - y
def mul(x, y):
return x * y
def div(x, y):
return x / y
def dispatch_func(operator, x, y):
return {
'add': add,
'sub': sub,
'mul': mul,
'div': div,
}.get(operator, lambda x, y: None)(x, y)
print(dispatch_func('add', 2, 8)) # 输出:10
```
4.使用try...except:
```python
def dispatch_try(operator, x, y):
try:
return {
'add': x + y,
'sub': x - y,
'mul': x * y,
'div': x / y,
}[operator]
except KeyError:
return None
print(dispatch_try('add', 2, 8)) # 输出:10
```
阅读全文
相关推荐
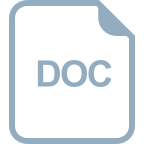
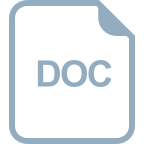
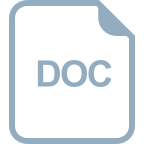















