编写一个文本加密程序,将英文文本文件in.txt加密成out.txt, 加密方法是A->Z,B -> Y,…,a -> z,b -> y,⋯z->a,其他字符不加密
时间: 2023-07-15 16:15:52 浏览: 99
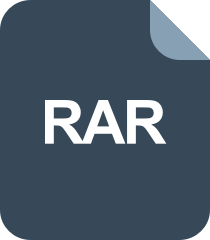
把文本信息加密到图片.rar_.dat加密图片_txt_图片加密_图片加密信息_文本加密
可以使用 Python 编写一个文本加密程序,代码如下:
```python
# 打开输入文件和输出文件
with open("in.txt", "r") as fin, open("out.txt", "w") as fout:
# 逐行读取输入文件
for line in fin:
# 对每个字符进行加密
encrypted_line = ""
for c in line:
if c.isalpha():
if c.isupper():
encrypted_line += chr(ord('Z') - ord(c) + ord('A'))
else:
encrypted_line += chr(ord('z') - ord(c) + ord('a'))
else:
encrypted_line += c
# 写入输出文件
fout.write(encrypted_line)
```
这个程序会将输入文件 `in.txt` 中的英文文本加密成输出文件 `out.txt`,加密方法是 A->Z, B->Y, ..., a->z, b->y, ..., z->a,其他字符不加密。可以根据实际需要修改输入文件和输出文件的文件名。
阅读全文
相关推荐
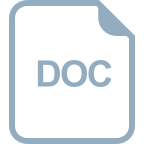
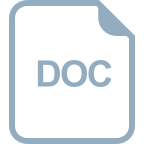





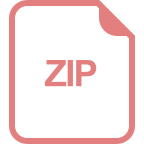
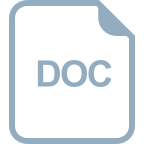
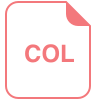
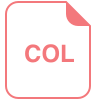
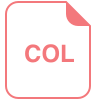
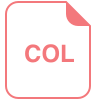
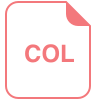
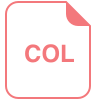


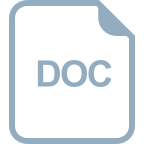