顺序表插入操作 #include<iostream> using namespace std; #
时间: 2024-09-17 20:05:07 浏览: 114
顺序表插入操作是指在顺序表的第i个位置插入元素e,并将原来位于第i个位置及其之后的元素都后移一个位置。具体实现可以使用一个循环将第i个位置及其之后的元素后移,然后将元素e插入到第i个位置。以下是一个C++实现的示例代码:
```
#include<iostream>
using namespace std;
#define OK 1
#define ERROR 0
#define MAXSIZE 100
typedef int datatype;
typedef struct {
datatype *elem;
int length;
} SqList;
int ListInsert_Sq(SqList &L, int i, datatype e) {
if ((i < 1) || (i > L.length + 1)) {
return ERROR;
}
if (L.length >= MAXSIZE) {
return ERROR;
}
for (int j = L.length; j >= i; j--) {
L.elem[j] = L.elem[j - 1];
}
L.elem[i - 1] = e;
L.length++;
return OK;
}
```
--相关问题--:
相关问题
顺序表删除重复元素 #include<iostream> using+namespace+std; #define+OK+1 #define+ERROR+0 #define+MAXSIZE+100 t
顺序表删除重复元素的步骤如下:
1. 首先,将顺序表中的第一个元素作为基准元素,从第二个元素开始遍历整个顺序表。
2. 如果遍历到的元素与基准元素相同,则将该元素删除。
3. 如果遍历到的元素与基准元素不同,则将该元素作为新的基准元素,继续遍历后面的元素。
4. 重复以上步骤,直到遍历完整个顺序表。
下面是具体的代码实现:
int DelRepeatElem(SqList &L)
{
int i, j, k;
if (L.length == 0) {
return ERROR;
}
for (i = 0; i < L.length; i++) {
k = L.data[i];
for (j = i + 1; j < L.length; j++) {
if (L.data[j] == k) {
L.length--;
L.data[j] = L.data[L.length];
j--;
}
}
}
return OK;
}
补充编程// map::find #include <iostream> #include <map> #include <string> #include <vector> using namespace std; void count(string str,int a[]){ } int main () { string str; cin>>str; int a[26]={0}; count(str,a); for(int i=0;i<26;i++) if(a[i]>0){ char ch='a'+i; cout<<ch<<" "<<a[i]<<endl; } return 0; }来完成任务:输入一串小写字母组成的字符串,按字母表顺序输出各个字母出现的次数。
你可以使用 `map` 来记录每个字母出现的次数,然后按照字母表顺序输出结果。下面是代码示例:
```c++
#include <iostream>
#include <map>
#include <string>
#include <vector>
using namespace std;
void count(string str, map<char, int>& m){
for(int i=0; i<str.length(); i++){
m[str[i]]++;
}
}
int main () {
string str;
cin>>str;
map<char, int> m;
count(str, m);
for(char ch='a'; ch<='z'; ch++){
if(m.find(ch) != m.end()){
cout<<ch<<" "<<m[ch]<<endl;
}
}
return 0;
}
```
在 `count` 函数中,我们遍历字符串 `str` 的每个字符,然后将其出现次数加一,保存在 map 中。在主函数中,我们遍历每个字母,如果它在 map 中出现过,就输出它的出现次数。注意,我们使用 `m.find(ch) != m.end()` 来判断某个字母是否出现过,因为如果它没有出现过,`m[ch]` 的值将是默认值0,这样就会误判。
阅读全文
相关推荐
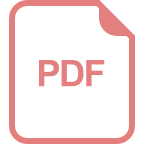
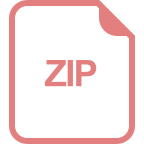














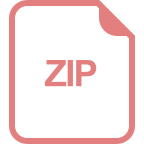
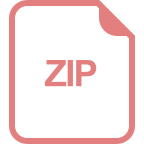