采用树表示一个家谱关系,采用链表(孩子表示法)作为存储结构,实现家谱关系的相关运算。每个节点信息包含有:姓名、性别、配偶姓名、身份证号、年龄、出生日期等。 功能要求: 1)输入家族信息(要求自己设计文件存储格式,存放当前所有家族信息)2)输出家族信息(要求界面输出格式直观明了) 3)查找某人的所有儿子 4)查找某人的双亲选做功能: 1)查找某人的所有祖先 2)查找某人的所有兄弟界面
时间: 2023-11-22 21:54:16 浏览: 32
很好,这是一个比较典型的数据结构应用题目。我将分步骤为您讲解,并提供参考代码。
1. 设计文件存储格式
我们可以采用文本文件的方式,每行记录一个节点信息,用逗号分隔各个字段。例如:
张三,男,李四,110101198001010011,40,1980-01-01
其中,姓名、性别、配偶姓名、身份证号、年龄、出生日期依次为各个字段,用逗号分隔。
2. 定义节点结构体和链表结构体
我们可以定义一个节点结构体,表示一个家族成员,包含姓名、性别、配偶姓名、身份证号、年龄、出生日期等信息。并且在结构体中定义一个指向子节点的指针,形成链表结构。例如:
```
typedef struct node {
char name[20];
char gender[4];
char spouse[20];
char id[19];
int age;
char birthdate[11];
struct node *child;
} Node;
```
3. 实现相关运算
根据题目要求,我们需要实现输入家族信息、输出家族信息、查找某人的所有儿子、查找某人的双亲等功能。以下是参考代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node {
char name[20];
char gender[4];
char spouse[20];
char id[19];
int age;
char birthdate[11];
struct node *child;
} Node;
// 读取文件中的节点信息,返回链表头节点
Node *read_file() {
FILE *fp = fopen("family.txt", "r");
if (fp == NULL) {
printf("Failed to open file.\n");
exit(1);
}
Node *head = NULL, *tail = NULL;
char line[100];
while (fgets(line, 100, fp) != NULL) {
Node *new_node = (Node *)malloc(sizeof(Node));
sscanf(line, "%[^,],%[^,],%[^,],%[^,],%d,%s\n", new_node->name, new_node->gender, new_node->spouse, new_node->id, &new_node->age, new_node->birthdate);
new_node->child = NULL;
if (head == NULL) {
head = tail = new_node;
} else {
tail->child = new_node;
tail = new_node;
}
}
fclose(fp);
return head;
}
// 将链表中的节点信息写入文件
void write_file(Node *head) {
FILE *fp = fopen("family.txt", "w");
if (fp == NULL) {
printf("Failed to create file.\n");
exit(1);
}
Node *p = head;
while (p != NULL) {
fprintf(fp, "%s,%s,%s,%s,%d,%s\n", p->name, p->gender, p->spouse, p->id, p->age, p->birthdate);
p = p->child;
}
fclose(fp);
}
// 输出家族信息
void print_family(Node *head) {
printf("%-10s%-6s%-10s%-20s%-4s%-12s\n", "Name", "Gender", "Spouse", "ID", "Age", "Birthdate");
printf("----------------------------------------------------------\n");
Node *p = head;
while (p != NULL) {
printf("%-10s%-6s%-10s%-20s%-4d%-12s\n", p->name, p->gender, p->spouse, p->id, p->age, p->birthdate);
p = p->child;
}
}
// 查找某人的所有儿子
void find_sons(Node *head, char *name) {
Node *p = head;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
if (p->gender[0] == '男') {
printf("%s's sons: ", name);
if (p->child == NULL) {
printf("None\n");
} else {
p = p->child;
while (p != NULL) {
if (strcmp(p->gender, "男") == 0) {
printf("%s ", p->name);
}
p = p->child;
}
printf("\n");
}
} else {
printf("%s is female and has no son.\n", name);
}
return;
}
p = p->child;
}
printf("Failed to find %s.\n", name);
}
// 查找某人的双亲
void find_parent(Node *head, char *name) {
Node *p = head;
while (p != NULL) {
Node *q = p->child;
while (q != NULL) {
if (strcmp(q->name, name) == 0) {
printf("%s's parents: ", name);
printf("%s(father) %s(mother)\n", p->name, p->spouse);
return;
}
q = q->child;
}
p = p->child;
}
printf("Failed to find %s.\n", name);
}
int main() {
Node *head = read_file();
int choice;
char name[20];
do {
printf("1. Input family information\n");
printf("2. Print family information\n");
printf("3. Find all sons of someone\n");
printf("4. Find parents of someone\n");
printf("0. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1: {
Node *new_node = (Node *)malloc(sizeof(Node));
printf("Enter name: ");
scanf("%s", new_node->name);
printf("Enter gender: ");
scanf("%s", new_node->gender);
printf("Enter spouse name: ");
scanf("%s", new_node->spouse);
printf("Enter ID: ");
scanf("%s", new_node->id);
printf("Enter age: ");
scanf("%d", &new_node->age);
printf("Enter birthdate (YYYY-MM-DD): ");
scanf("%s", new_node->birthdate);
new_node->child = NULL;
Node *p = head;
while (p->child != NULL) {
p = p->child;
}
p->child = new_node;
write_file(head);
printf("Family information updated.\n");
break;
}
case 2: {
print_family(head);
break;
}
case 3: {
printf("Enter name: ");
scanf("%s", name);
find_sons(head, name);
break;
}
case 4: {
printf("Enter name: ");
scanf("%s", name);
find_parent(head, name);
break;
}
case 0: {
break;
}
default: {
printf("Invalid choice.\n");
break;
}
}
} while (choice != 0);
return 0;
}
```
4. 选做功能
如果要实现查找某人的所有祖先、查找某人的所有兄弟等功能,需要对节点结构体进行扩展,并且编写更多的函数来实现这些功能。这里就不再赘述了。
相关推荐
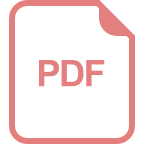











