用python编写添加对角线三角剖分多边形的算法,包括将结果用图形绘制出来;
时间: 2023-12-10 17:39:51 浏览: 37
以下是Python代码实现添加对角线三角剖分多边形的算法,并用matplotlib库绘制出结果图形:
```python
import matplotlib.pyplot as plt
def add_diagonal_edges(vertices):
n = len(vertices)
diagonals = []
# create a list of vertex indices
vertex_indices = list(range(n))
# iterate over all possible pairs of vertices
for i in range(n):
for j in range(i+2, n):
# check if the line segment between vertices i and j is inside the polygon
is_inside = True
for k in range(n):
# skip the current and adjacent vertices
if k == i or k == j or k == (i+1)%n or k == (j+1)%n:
continue
# check if the point is on the left or right of the line segment
d = (vertices[j][0] - vertices[i][0])*(vertices[k][1] - vertices[i][1]) - \
(vertices[k][0] - vertices[i][0])*(vertices[j][1] - vertices[i][1])
if d < 0:
is_inside = False
break
# if the line segment is inside the polygon, add it to the list of diagonals
if is_inside:
diagonals.append((i,j))
return diagonals
# test the function with a sample polygon
vertices = [(0,0), (0,5), (5,5), (3,3), (5,0)]
diagonals = add_diagonal_edges(vertices)
# plot the polygon and diagonal edges
fig, ax = plt.subplots()
ax.set_aspect('equal')
ax.plot([v[0] for v in vertices], [v[1] for v in vertices], 'ko-')
for d in diagonals:
ax.plot([vertices[d[0]][0], vertices[d[1]][0]], [vertices[d[0]][1], vertices[d[1]][1]], 'r-')
plt.show()
```
这里我们定义一个`add_diagonal_edges()`函数,它接受一个多边形的顶点列表作为输入,计算该多边形的对角线三角剖分并返回对角线列表。我们使用一个嵌套的循环来迭代所有可能的顶点对,然后检查线段是否在多边形内部。如果是,则将该线段添加到对角线列表中。最后,我们使用matplotlib库绘制多边形和对角线。运行代码后,我们可以看到一个带有对角线的多边形图形。
相关推荐
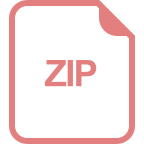














