C语言用递归方法求解n皇后
时间: 2023-10-22 09:06:50 浏览: 88
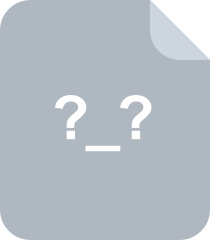
用递归方法求解n皇后问题
以下是用C语言递归方法求解n皇后问题的代码:
```c
#include <stdio.h>
#include <stdlib.h>
int n = 8; // 棋盘大小
int count = 0; // 解的个数
void print_solution(int *solution) {
printf("Solution %d:\n", count);
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if (j == solution[i]) {
printf("Q ");
} else {
printf(". ");
}
}
printf("\n");
}
}
// 判断当前位置是否合法
int is_valid(int *solution, int row, int col) {
for (int i = 0; i < row; i++) {
if (solution[i] == col || abs(row - i) == abs(col - solution[i])) {
return 0;
}
}
return 1;
}
void n_queens(int *solution, int row) {
if (row == n) { // 找到一个解
count++;
print_solution(solution);
return;
}
for (int col = 0; col < n; col++) {
if (is_valid(solution, row, col)) {
solution[row] = col;
n_queens(solution, row + 1);
}
}
}
int main() {
int *solution = (int *) malloc(n * sizeof(int));
n_queens(solution, 0);
printf("Total solutions: %d\n", count);
free(solution);
return 0;
}
```
在这个代码中,`n`表示棋盘大小,`count`表示解的个数。`print_solution`函数用于打印一组解,`is_valid`函数用于判断当前位置是否合法。`n_queens`函数是递归求解n皇后问题的核心函数。它的参数`solution`表示当前已经找到的部分解,`row`表示当前正在处理第几行。在每一行中,枚举每一列并检查当前位置是否合法。如果合法,则把当前位置加入部分解,并递归处理下一行。如果已经处理完所有行,则找到了一个解,打印出来,并返回。最后,在`main`函数中调用`n_queens`函数,并输出解的个数。
阅读全文
相关推荐




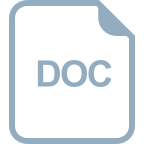
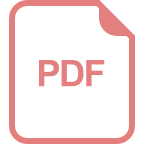








