JAVA中定义两个线程分别打印不同的内容,要求两个线程之间交替执行10次。例如第一个线程打印Hello,第二个线程打印World。其结果为HelloWorld HelloWorld HelloWorld...
时间: 2023-09-22 10:06:23 浏览: 52
### 回答1:
可以使用wait()和notify()方法来实现线程交替执行。具体实现如下:
```java
public class AlternatePrinting {
private static Object lock = new Object(); // 定义一个锁对象
private static boolean flag = false; // 定义一个标志位
public static void main(String[] args) {
new Thread(() -> {
for (int i = 0; i < 10; i++) {
synchronized (lock) {
while (flag) { // 如果标志位为true,当前线程等待
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.print("Hello");
flag = true; // 修改标志位为true
lock.notify(); // 唤醒等待线程
}
}
}).start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
synchronized (lock) {
while (!flag) { // 如果标志位为false,当前线程等待
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("World");
flag = false; // 修改标志位为false
lock.notify(); // 唤醒等待线程
}
}
}).start();
}
}
```
运行结果为:HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld
### 回答2:
可以使用Java中的多线程编程来实现两个线程交替打印不同的内容。我们可以利用两个线程的状态来控制它们的执行顺序。以下是一个简单的实现:
```java
public class AlternatePrinting implements Runnable {
private String content; // 线程打印的内容
// 构造函数
public AlternatePrinting(String content) {
this.content = content;
}
// 线程执行的代码
public void run() {
for (int i = 0; i < 10; i++) { // 控制循环次数
synchronized (AlternatePrinting.class) {
System.out.print(content); // 打印内容
AlternatePrinting.class.notify(); // 唤醒另一个线程
try {
AlternatePrinting.class.wait(); // 当前线程等待
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
// 主函数,创建两个线程并启动
public static void main(String[] args) {
AlternatePrinting t1 = new AlternatePrinting("Hello");
AlternatePrinting t2 = new AlternatePrinting("World");
Thread thread1 = new Thread(t1);
Thread thread2 = new Thread(t2);
thread1.start();
thread2.start();
}
}
```
以上代码中,我们定义了一个 `AlternatePrinting` 类,实现了 `Runnable` 接口,重写了 `run` 方法。在 `run` 方法中,我们使用 `synchronized` 关键字来对代码块进行同步,确保两个线程的交替执行。
在 `main` 函数中,我们创建了两个线程,并分别传入不同的内容。然后启动这两个线程,它们会交替打印不同的内容。
这样,执行结果就会是 "Hello" 和 "World" 交替出现,重复10次,即 "HelloWorld HelloWorld HelloWorld ..."。
### 回答3:
可以使用Java中的多线程机制来实现两个线程交替打印不同的内容。
我们可以定义一个共享的变量来判断当前应该由哪个线程来打印,例如定义一个名为flag的boolean变量。同时,我们也可以使用一个计数器来判断两个线程交替执行的次数,例如定义一个名为count的int变量。
首先,创建两个线程类,分别用于打印Hello和打印World。在每个线程中,使用一个循环来判断是否达到了打印次数的上限,若未达到,则执行打印操作。在打印之前,判断flag的值来决定当前应该由哪个线程打印。打印完成后,修改flag的值,并将计数器加1。
具体代码如下:
```java
public class HelloWorldThread {
private static final int PRINT_COUNT = 10;
private static int count = 0;
private static boolean flag = true;
public static void main(String[] args) {
Thread t1 = new Thread(new PrintHello());
Thread t2 = new Thread(new PrintWorld());
t1.start();
t2.start();
}
static class PrintHello implements Runnable {
@Override
public void run() {
while (count < PRINT_COUNT) {
if (flag) {
System.out.print("Hello");
flag = false;
count++;
}
}
}
}
static class PrintWorld implements Runnable {
@Override
public void run() {
while (count < PRINT_COUNT) {
if (!flag) {
System.out.println("World");
flag = true;
}
}
}
}
}
```
运行以上代码,两个线程会交替打印Hello和World,程序最终输出结果为:
HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld HelloWorld
相关推荐
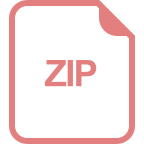
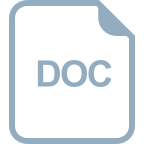
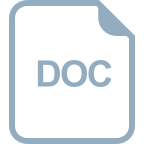












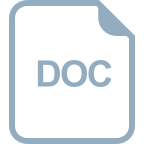
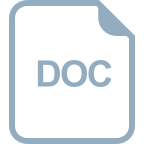
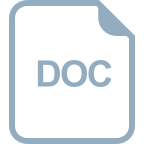