micropython多线程显示
时间: 2023-05-20 21:00:58 浏览: 119
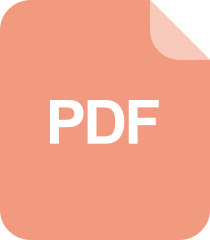
python多线程
Micropython支持多线程,可以使用_thread模块来实现。以下是一个简单的示例代码,可以在Micropython中使用多线程显示:
```python
import _thread
import time
def display_thread():
while True:
print("Hello from display thread!")
time.sleep(1)
_thread.start_new_thread(display_thread, ())
while True:
print("Hello from main thread!")
time.sleep(1)
```
这个代码创建了两个线程,一个是主线程,另一个是显示线程。显示线程每秒钟输出一次“Hello from display thread!”,主线程每秒钟输出一次“Hello from main thread!”。可以看到,两个线程交替执行,实现了多线程显示的效果。
阅读全文
相关推荐




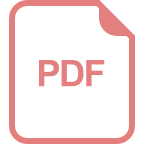
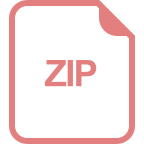
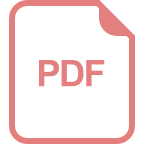
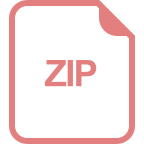
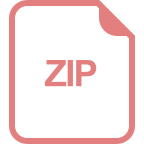
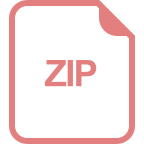
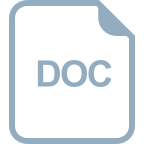
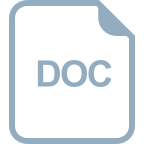
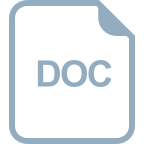
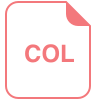
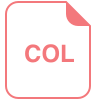
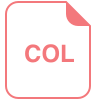