解释 final_adv_imgs = final_adv_imgs[:, :, ::-1].transpose(1, 2, 0) # 将RGB通道顺序变为BGR通道顺序 ValueError: axes don't match array
时间: 2024-02-25 11:51:42 浏览: 122
这段代码中的错误与np.transpose函数的使用方式有关。根据错误提示信息,报错是因为传递给np.transpose函数的第二个参数(即轴的顺序)不符合数组的维度。具体来说,final_adv_imgs的维度应该是HWC(高度x宽度x通道数),但是传递给np.transpose函数的第二个参数却是(1, 2, 0),这个参数表示将final_adv_imgs的第二个维度放到转置后数组的第一个维度,将第三个维度放到第二个维度,将第一个维度放到第三个维度。这样的顺序是错误的,应该将第三个维度放到第一个维度,将第一和第二个维度交换位置,即使用(2, 0, 1)作为第二个参数。修改后的代码如下:
```
final_adv_imgs = final_adv_imgs[:, :, ::-1].transpose(2, 0, 1) # 将RGB通道顺序变为BGR通道顺序
```
这个修改后的代码将RGB通道顺序变为BGR通道顺序,并且使用transpose函数将final_adv_imgs的维度从HWC转换为CHW。这样的操作通常用于深度学习计算,因为大多数深度学习框架使用的是CHW格式的图像数据。
相关问题
def adversarial(x, model, loss_func, c=1e-4, kappa=0, num_iter=100, lr=0.01): """ Create adversarial examples using CW algorithm Args: - x: input image - model: the neural network model - loss_func: the loss function to use - c: the weight for the L2 regularization term (default=1e-4) - kappa: the confidence parameter (default=0) - num_iter: number of iterations for the algorithm (default=100) - lr: learning rate for the optimization (default=0.01) Returns: - x_adv: adversarial example """ x_adv = x.clone().detach().requires_grad_(True) for i in range(num_iter): output = model(x_adv) loss = loss_func(output, torch.tensor([kappa]), x, x_adv, c) model.zero_grad() loss.backward() with torch.no_grad(): x_adv += lr * x_adv.grad x_adv = torch.max(torch.min(x_adv, x + 0.35), x - 0.35) x_adv = torch.clamp(x_adv, 0, 1) x_adv.requires_grad_() return x_adv.detach()上述代码出现TypeError: ce_loss() takes 2 positional arguments but 5 were given错误,请改正
根据代码,`loss_func`应该是一个函数,且它的输入参数应该是模型的输出 `output`和目标标签 `target`。因此,需要修改 `loss_func` 函数的输入参数,将 `x`、`x_adv` 和 `c` 作为函数的参数,而不是在函数调用时传入。修改后的代码如下:
```
def adversarial(x, model, loss_func, c=1e-4, kappa=0, num_iter=100, lr=0.01):
"""Create adversarial examples using CW algorithm
Args:
- x: input image
- model: the neural network model
- loss_func: the loss function to use
- c: the weight for the L2 regularization term (default=1e-4)
- kappa: the confidence parameter (default=0)
- num_iter: number of iterations for the algorithm (default=100)
- lr: learning rate for the optimization (default=0.01)
Returns:
- x_adv: adversarial example
"""
x_adv = x.clone().detach().requires_grad_(True)
for i in range(num_iter):
output = model(x_adv)
loss = loss_func(output, kappa, target=x, adversary=x_adv, c=c)
model.zero_grad()
loss.backward()
with torch.no_grad():
x_adv += lr * x_adv.grad
x_adv = torch.max(torch.min(x_adv, x + 0.35), x - 0.35)
x_adv = torch.clamp(x_adv, 0, 1)
x_adv.requires_grad_()
return x_adv.detach()
```
def FGSM(self, x, y_true, y_target=None, eps=0.03, alpha=2/255, iteration=1): self.set_mode('eval') x = Variable(cuda(x, self.cuda), requires_grad=True) y_true = Variable(cuda(y_true, self.cuda), requires_grad=False) if y_target is not None: targeted = True y_target = Variable(cuda(y_target, self.cuda), requires_grad=False) else: targeted = False h = self.net(x) prediction = h.max(1)[1] accuracy = torch.eq(prediction, y_true).float().mean() cost = F.cross_entropy(h, y_true) if iteration == 1: if targeted: x_adv, h_adv, h = self.attack.fgsm(x, y_target, True, eps) else: x_adv, h_adv, h = self.attack.fgsm(x, y_true, False, eps) else: if targeted: x_adv, h_adv, h = self.attack.i_fgsm(x, y_target, True, eps, alpha, iteration) else: x_adv, h_adv, h = self.attack.i_fgsm(x, y_true, False, eps, alpha, iteration) prediction_adv = h_adv.max(1)[1] accuracy_adv = torch.eq(prediction_adv, y_true).float().mean() cost_adv = F.cross_entropy(h_adv, y_true) # make indication of perturbed images that changed predictions of the classifier if targeted: changed = torch.eq(y_target, prediction_adv) else: changed = torch.eq(prediction, prediction_adv) changed = torch.eq(changed, 0) changed = changed.float().view(-1, 1, 1, 1).repeat(1, 3, 28, 28) changed[:, 0, :, :] = where(changed[:, 0, :, :] == 1, 252, 91) changed[:, 1, :, :] = where(changed[:, 1, :, :] == 1, 39, 252) changed[:, 2, :, :] = where(changed[:, 2, :, :] == 1, 25, 25) changed = self.scale(changed/255) changed[:, :, 3:-2, 3:-2] = x_adv.repeat(1, 3, 1, 1)[:, :, 3:-2, 3:-2] self.set_mode('train') return x_adv.data, changed.data,\ (accuracy.item(), cost.item(), accuracy_adv.item(), cost_adv.item())
这段代码是一个实现了FGSM(Fast Gradient Sign Method)攻击的函数。FGSM是一种用于生成对抗样本的方法,通过在输入样本上添加一小步扰动来欺骗分类器,使其产生错误的分类结果。
该函数的输入参数包括原始输入样本x、真实标签y_true、目标标签y_target(可选)、扰动范围eps、扰动步长alpha和迭代次数iteration。
函数首先将模型设置为评估模式,然后将输入样本转化为可求导变量,并计算原始样本在模型上的预测结果和准确率。然后计算原始样本在模型上的交叉熵损失。
接下来根据迭代次数选择使用FGSM攻击还是I-FGSM(Iterative FGSM)攻击。如果目标标签y_target存在,则使用目标攻击,否则使用非目标攻击。攻击过程中,对输入样本添加扰动,并计算扰动后的样本在模型上的预测结果和准确率。
最后,将产生的对抗样本、扰动图像、原始样本在模型上的准确率和损失作为输出返回。
值得注意的是,代码中还包含了一个用于生成扰动图像的部分。首先根据模型预测结果确定哪些样本的分类结果发生了变化,然后将这些样本的对应像素位置修改为特定的颜色值,以突出显示扰动部分。最后将扰动图像与对抗样本叠加,形成最终的扰动图像。
请问我还能为您解答其他问题吗?
阅读全文
相关推荐
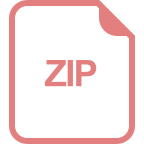
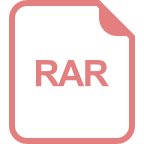
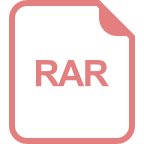
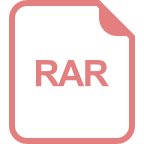
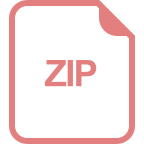
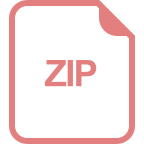
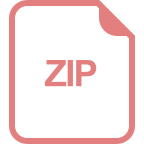
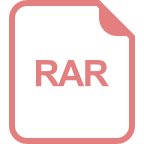
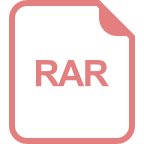
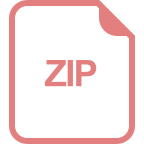







