批量人像彩色化的算法代码
时间: 2023-10-05 17:13:35 浏览: 37
以下是一个基于深度学习的批量人像彩色化算法的代码示例。这个算法使用了基于 PyTorch 框架的 Colorful Image Colorization 模型。
```python
import os
import cv2
import numpy as np
import torch
import torchvision.transforms as transforms
from model import ColorfulColorization
# 加载模型
model = ColorfulColorization()
model.load_state_dict(torch.load('model.pth', map_location=torch.device('cpu')))
model.eval()
# 转换图像
def transform(image):
transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize(mean=[0.5, 0.5, 0.5],
std=[0.5, 0.5, 0.5])
])
return transform(image)
# 批量处理
def batch_process(input_dir, output_dir):
for filename in os.listdir(input_dir):
if not filename.endswith('.jpg'):
continue
# 加载图像
image = cv2.imread(os.path.join(input_dir, filename))
image_lab = cv2.cvtColor(image, cv2.COLOR_BGR2LAB)
image_l = image_lab[:, :, 0]
# 预测
image_l = transform(image_l)
with torch.no_grad():
image_ab = model(image_l.unsqueeze(0))[0]
image_ab = image_ab.cpu().numpy().transpose((1, 2, 0))
image_ab = cv2.resize(image_ab, (image.shape[1], image.shape[0]))
# 转换到 BGR 颜色空间
image_ab = cv2.cvtColor(image_ab, cv2.COLOR_LAB2BGR)
image_ab = np.clip(image_ab, 0, 1)
# 合并 L 和 AB 通道
image_lab = np.zeros((image.shape[0], image.shape[1], 3), dtype=np.uint8)
image_lab[:, :, 0] = image_l.numpy()[0] * 255
image_lab[:, :, 1:] = image_ab * 255
image_bgr = cv2.cvtColor(image_lab, cv2.COLOR_LAB2BGR)
# 保存输出图像
output_filename = os.path.join(output_dir, filename)
cv2.imwrite(output_filename, image_bgr)
# 运行批量处理
input_dir = 'input'
output_dir = 'output'
batch_process(input_dir, output_dir)
```
在这个例子中,我们首先加载了 PyTorch 模型并定义了一个图像转换函数。然后,我们使用 OpenCV 库来读取和处理输入图像。我们使用 `ColorfulColorization` 模型来预测图像的 AB 通道,并将其转换回 BGR 颜色空间。最后,我们将图像的 L 和 AB 通道合并,并将其转换回 BGR 颜色空间,然后保存输出图像。您需要将上述代码保存为一个 Python 文件,然后将输入图像放入 `input` 文件夹中,输出图像将保存在 `output` 文件夹中。您还需要从 [GitHub](https://github.com/richzhang/colorization/blob/master/colorization/models/colorization_deploy_v2.prototxt) 下载模型文件,并将其保存为 `model.pth`。
相关推荐
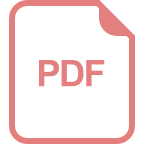
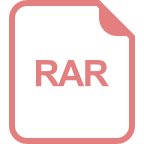
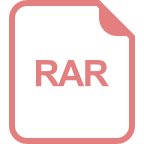














