C++数据结构 线性结构 (10,20,30,40,50,60,70,80,90,100)编写顺序表存储,实现以下操作:。 (1)查找第20个数据元素。 (2)查找数据元素100 (3 )删除一个数据元素50 (4)打印表的长度,以及表中所有元素。
时间: 2024-09-29 10:16:18 浏览: 32
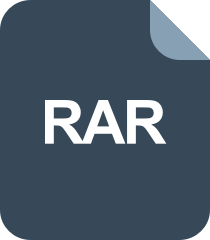
顺序表C++_数据结构实验--顺序表;C++语言_
线性结构是一种最基础的数据结构,它将数据项按照一定的顺序排列,每个数据项可以看作是一个节点,通过链接的方式连接起来形成一条链。对于给定的序列(10, 20, 30, 40, 50, 60, 70, 80, 90, 100),我们可以创建一个简单的顺序表(也称为数组)来存储这些元素。这里使用C++实现:
```cpp
#include <iostream>
using namespace std;
// 定义顺序表节点
struct ListNode {
int data;
ListNode* next;
};
class SequentialList {
private:
ListNode* head; // 链表头指针
int size; // 表长
public:
SequentialList() : head(nullptr), size(0) {}
// 添加元素到列表末尾
void add(int value) {
ListNode* newNode = new ListNode{value, nullptr};
if (head == nullptr) {
head = newNode;
} else {
ListNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
size++;
}
// 查找指定索引位置的数据
int find(int index) {
if (index >= 0 && index < size) {
ListNode* current = head;
for (int i = 0; i < index; ++i) {
current = current->next;
}
return current->data;
} else {
throw out_of_range("Index is out of range");
}
}
// 删除指定值的数据元素
void remove(int value) {
if (head == nullptr) return;
if (head->data == value) {
ListNode* temp = head;
head = head->next;
delete temp;
size--;
} else {
ListNode* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
ListNode* temp = current->next;
current->next = current->next->next;
delete temp;
size--;
}
}
}
// 打印表的长度和所有元素
void print() {
ListNode* current = head;
cout << "Length: " << size << endl;
while (current != nullptr) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
// 主函数演示上述操作
static void mainExample() {
SequentialList list;
for (int i = 10; i <= 100; i += 10) {
list.add(i);
}
// 操作演示
cout << "Find element at index 20: " << list.find(20) << endl;
cout << "Find element 100: " << list.find(100) << endl;
list.remove(50);
list.print();
}
};
int main() {
SequentialList::mainExample();
return 0;
}
```
这个示例展示了如何使用顺序表数据结构实现查找、删除和打印功能,并在`mainExample()`函数中演示了操作过程。
阅读全文
相关推荐
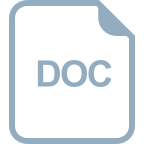
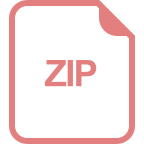


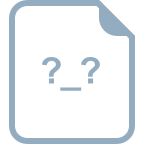
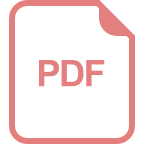
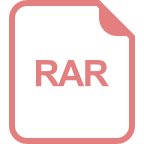
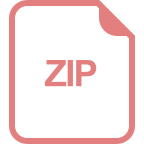
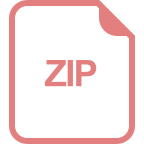
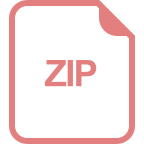
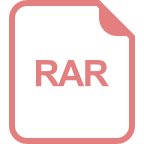
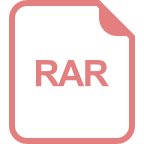
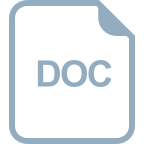
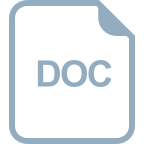
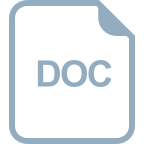
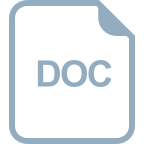
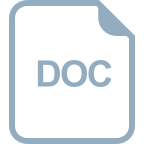