帮我写一段代码,实现将filter查询数据库结果,使用ajax渲染到前端
时间: 2023-12-28 22:06:21 浏览: 25
好的,假设你正在使用Python Flask框架和MySQL数据库。以下是一个简单的例子,演示如何使用Ajax从前端发送过滤查询,然后将结果渲染到前端。
1. 创建一个HTML模板,包含一个文本框和一个按钮:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Filter Results</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1>Filter Results</h1>
<input type="text" id="filter_input">
<button id="filter_button">Filter</button>
<table id="result_table">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody></tbody>
</table>
<script>
$(document).ready(function() {
$("#filter_button").click(function() {
var filter = $("#filter_input").val();
$.ajax({
url: "/filter",
type: "POST",
data: {filter: filter},
success: function(data) {
$("#result_table tbody").html(data);
}
});
});
});
</script>
</body>
</html>
```
2. 创建一个Flask路由,处理filter查询并返回渲染模板:
```python
from flask import Flask, render_template, request
import mysql.connector
app = Flask(__name__)
# MySQL连接配置
config = {
"user": "root",
"password": "",
"host": "127.0.0.1",
"database": "test_db"
}
@app.route("/")
def index():
return render_template("filter.html")
@app.route("/filter", methods=["POST"])
def filter_results():
filter = request.form["filter"]
# 连接到MySQL数据库
cnx = mysql.connector.connect(**config)
cursor = cnx.cursor()
# 执行过滤查询
query = "SELECT id, name, age FROM users WHERE name LIKE '%{}%'".format(filter)
cursor.execute(query)
# 获取结果
rows = cursor.fetchall()
# 关闭数据库连接
cursor.close()
cnx.close()
# 渲染HTML模板
return render_template("result.html", rows=rows)
```
3. 创建一个Flask模板,渲染filter查询结果:
```html
{% for row in rows %}
<tr>
<td>{{ row[0] }}</td>
<td>{{ row[1] }}</td>
<td>{{ row[2] }}</td>
</tr>
{% endfor %}
```
4. 运行Flask应用程序并在浏览器中访问:http://localhost:5000/
这样就可以在前端输入过滤条件,然后通过Ajax将查询结果渲染到前端页面中。
相关推荐
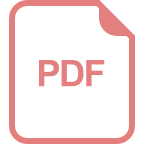
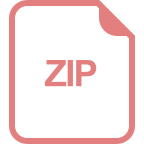














