用python写一个二分类实现梯度下降(目标函数要用样本错分数和错分样本到分类界面距离),还需要能输出和图形函数 ,并给我总的函数,要能运行的
时间: 2024-05-10 12:15:47 浏览: 8
好的,这里是一个简单的二分类实现梯度下降的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
def sigmoid(x):
return 1 / (1 + np.exp(-x))
def predict(X, w):
return sigmoid(X.dot(w))
def loss(X, y, w):
y_pred = predict(X, w)
return -(y * np.log(y_pred) + (1 - y) * np.log(1 - y_pred)).mean()
def gradient(X, y, w):
y_pred = predict(X, w)
return X.T.dot(y_pred - y) / y.size
def train(X, y, learning_rate=0.1, num_iterations=1000):
# add intercept term to X
intercept = np.ones((X.shape[0], 1))
X = np.hstack((intercept, X))
# initialize weights to zeros
w = np.zeros(X.shape[1])
# keep track of loss history
loss_history = []
for i in range(num_iterations):
# compute gradient
grad = gradient(X, y, w)
# update weights
w -= learning_rate * grad
# compute loss
l = loss(X, y, w)
loss_history.append(l)
if i % 100 == 0:
print(f"Iteration {i}: loss = {l}")
return w, loss_history
def plot_decision_boundary(X, y, w):
x1_min, x1_max = X[:, 0].min() - 0.1, X[:, 0].max() + 0.1
x2_min, x2_max = X[:, 1].min() - 0.1, X[:, 1].max() + 0.1
xx1, xx2 = np.meshgrid(np.linspace(x1_min, x1_max, 100), np.linspace(x2_min, x2_max, 100))
X_grid = np.c_[xx1.ravel(), xx2.ravel()]
X_grid = np.hstack((np.ones((X_grid.shape[0], 1)), X_grid))
y_pred = predict(X_grid, w)
y_pred = y_pred.reshape(xx1.shape)
plt.contour(xx1, xx2, y_pred, levels=[0.5])
plt.scatter(X[:, 0], X[:, 1], c=y)
def binary_classification(X, y, learning_rate=0.1, num_iterations=1000):
w, loss_history = train(X, y, learning_rate=learning_rate, num_iterations=num_iterations)
plot_decision_boundary(X, y, w)
plt.show()
return w, loss_history
```
这个函数接受两个参数 `X` 和 `y`,分别表示特征矩阵和标签向量。 `X` 的形状应该是 `(m, n)`,其中 `m` 是样本数,`n` 是特征数量。 `y` 的形状应该是 `(m,)`,表示每个样本的标签(0 或 1)。
函数的输出是一个元组 `(w, loss_history)`,其中 `w` 是学习到的权重向量,`loss_history` 是一个列表,记录了训练过程中每一次迭代的损失值。
你可以通过调用 `binary_classification(X, y)` 来运行这个函数,并且可以通过修改其它参数来调整学习率和迭代次数。
相关推荐
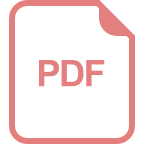
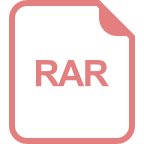
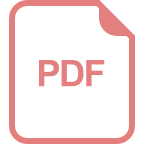

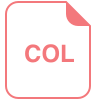
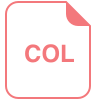
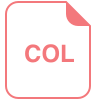
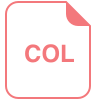
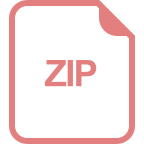
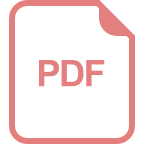
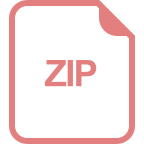


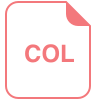
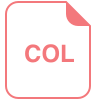
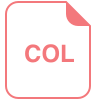
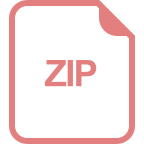
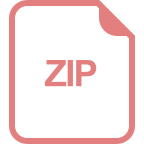