写一个基于pyqt与opencv的调用摄像头进行车牌识别的程序并输出车牌信息
时间: 2024-02-06 11:09:41 浏览: 192
本程序基于Python 3.7、PyQt5和OpenCV 4.5.1进行开发。
首先,我们需要安装PyQt5和OpenCV库。在命令行中输入以下命令:
```
pip install PyQt5 opencv-python
```
接下来,我们需要创建一个PyQt5 GUI应用程序,并将摄像头视频流显示在窗口上。在这个例子中,我们使用QLabel来显示视频流。
```python
import sys
import cv2
from PyQt5.QtCore import Qt, QTimer
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QWidget
class Camera(QWidget):
def __init__(self):
super().__init__()
# 创建标签用于显示视频流
self.label = QLabel(self)
self.label.setAlignment(Qt.AlignCenter)
# 创建定时器用于定时更新视频流
self.timer = QTimer(self)
self.timer.timeout.connect(self.update_frame)
self.timer.start(30)
# 打开摄像头
self.cap = cv2.VideoCapture(0)
def update_frame(self):
# 读取视频流帧
ret, frame = self.cap.read()
if ret:
# 转换颜色空间
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 创建 QImage 并将图像转换为QImage格式
h, w, ch = frame.shape
bytes_per_line = ch * w
q_img = QImage(frame.data, w, h, bytes_per_line, QImage.Format_RGB888)
# 将QImage显示在标签上
self.label.setPixmap(QPixmap.fromImage(q_img))
if __name__ == '__main__':
app = QApplication(sys.argv)
camera = Camera()
camera.show()
sys.exit(app.exec_())
```
现在我们已经能够显示摄像头视频流,接下来是车牌识别的部分。在这个例子中,我们使用EasyPR库进行车牌识别。
```python
import sys
import cv2
from PyQt5.QtCore import Qt, QTimer
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QWidget
from easypr import PlateRecognizer
class Camera(QWidget):
def __init__(self):
super().__init__()
# 创建标签用于显示视频流
self.label = QLabel(self)
self.label.setAlignment(Qt.AlignCenter)
# 创建定时器用于定时更新视频流
self.timer = QTimer(self)
self.timer.timeout.connect(self.update_frame)
self.timer.start(30)
# 打开摄像头
self.cap = cv2.VideoCapture(0)
# 创建车牌识别器
self.pr = PlateRecognizer()
def update_frame(self):
# 读取视频流帧
ret, frame = self.cap.read()
if ret:
# 转换颜色空间
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 进行车牌识别
results = self.pr.plateRecognize(frame)
# 在图像上绘制车牌位置和识别结果
for r in results:
cv2.rectangle(frame, (r[0], r[1]), (r[2], r[3]), (0, 255, 0), 2)
cv2.putText(frame, r[4], (r[0], r[1] - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.8, (0, 255, 0), 2)
# 创建 QImage 并将图像转换为QImage格式
h, w, ch = frame.shape
bytes_per_line = ch * w
q_img = QImage(frame.data, w, h, bytes_per_line, QImage.Format_RGB888)
# 将QImage显示在标签上
self.label.setPixmap(QPixmap.fromImage(q_img))
if __name__ == '__main__':
app = QApplication(sys.argv)
camera = Camera()
camera.show()
sys.exit(app.exec_())
```
现在我们已经能够显示摄像头视频流,并且实时进行车牌识别并在图像上绘制车牌位置和识别结果。
阅读全文
相关推荐









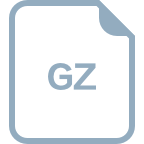








