写一个基于pyqt与opencv的调用摄像头识别中文车牌并输出车牌信息的程序
时间: 2023-12-16 15:04:09 浏览: 166
好的,这是一个简单的基于PyQt和OpenCV的车牌识别程序。需要注意的是,本程序只能识别中文车牌,对于其他语言的车牌可能会出现错误。
```python
import sys
import cv2
import numpy as np
from PyQt5.QtCore import Qt, QTimer, QRect
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QVBoxLayout, QHBoxLayout
# 定义车牌识别模型路径
cascade_path = "haarcascade_chinese_plate_number.xml"
class VideoLabel(QLabel):
def __init__(self):
super().__init__()
self.setFixedSize(640, 480) # 设置标签大小
self.setAlignment(Qt.AlignCenter) # 设置文字居中显示
def setImage(self, image):
self.setPixmap(QPixmap.fromImage(image)) # 将图像转为QPixmap并显示在标签上
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("车牌识别程序")
self.setFixedSize(640, 600)
# 初始化界面
self.initUI()
# 初始化摄像头
self.camera = cv2.VideoCapture(0)
# 初始化定时器
self.timer = QTimer()
self.timer.timeout.connect(self.updateImage)
self.timer.start(30)
def initUI(self):
# 创建标签
self.video_label = VideoLabel()
self.plate_label = QLabel("车牌号码:")
# 创建布局
vbox = QVBoxLayout()
vbox.addWidget(self.video_label)
vbox.addWidget(self.plate_label)
hbox = QHBoxLayout()
hbox.addStretch()
hbox.addLayout(vbox)
hbox.addStretch()
# 设置布局
self.setLayout(hbox)
def updateImage(self):
# 读取摄像头图像
ret, frame = self.camera.read()
# 将图像转为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 加载车牌识别模型
cascade = cv2.CascadeClassifier(cascade_path)
# 识别车牌
plates = cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=3, minSize=(30, 30), flags=cv2.CASCADE_SCALE_IMAGE)
# 标注车牌
for (x, y, w, h) in plates:
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 裁剪车牌区域并转换为二值图像
plate = gray[y:y + h, x:x + w]
plate = cv2.threshold(plate, 0, 255, cv2.THRESH_BINARY_INV | cv2.THRESH_OTSU)[1]
# 识别车牌号码
text = pytesseract.image_to_string(plate, lang='chi_sim', config='--psm 7')
# 显示车牌号码
self.plate_label.setText("车牌号码:" + text)
# 将图像转为Qt图像并显示在标签上
image = QImage(frame, frame.shape[1], frame.shape[0], QImage.Format_RGB888)
self.video_label.setImage(image)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
需要安装的库:PyQt5、opencv-python、pytesseract
运行程序后,摄像头会自动启动并显示在窗口中,如果有车牌出现,程序会自动识别车牌并在窗口下方显示车牌号码。
阅读全文
相关推荐
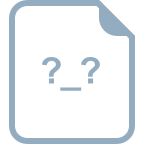
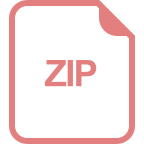
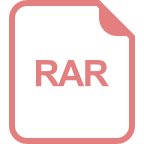















