c语言将链表反向连接
时间: 2024-01-06 14:03:49 浏览: 27
要将链表反向连接,可以使用三个指针来完成操作。首先,将当前节点的下一个节点保存在一个临时指针中,然后将当前节点的下一个节点指向前一个节点,最后将前一个节点指向当前节点。重复这个过程直到遍历完整个链表。最后,将最后一个节点设置为新的头节点。
以下是一个示例代码来演示如何在C语言中将链表反向连接:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
struct Node {
int data;
struct Node* next;
};
// 反向连接链表函数
struct Node* reverseLinkedList(struct Node* head) {
struct Node* prev = NULL;
struct Node* current = head;
struct Node* next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
head = prev;
return head;
}
// 打印链表函数
void printLinkedList(struct Node* head) {
struct Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
// 主函数
int main() {
// 创建链表
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
struct Node* second = (struct Node*)malloc(sizeof(struct Node));
struct Node* third = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
printf("原始链表:");
printLinkedList(head);
// 反向连接链表
head = reverseLinkedList(head);
printf("反向连接后的链表:");
printLinkedList(head);
return 0;
}
```
相关推荐
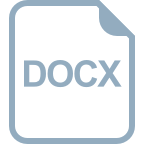














