io模拟spi代码 stm32
时间: 2023-07-09 11:29:26 浏览: 184
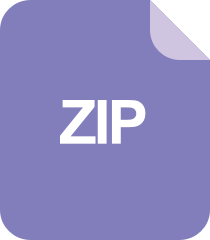
模拟i2c.zip_STM32F103_i2c stm32 io模拟_spi_stm32 模拟_模拟I2C
以下是一个简单的使用STM32的SPI接口进行IO模拟的代码示例:
```c
#include "stm32f4xx.h"
// 定义SPI接口的引脚
#define SPI_GPIO_PORT GPIOB
#define SPI_GPIO_CLK RCC_AHB1Periph_GPIOB
#define SPI_PIN_CLK GPIO_Pin_13
#define SPI_PIN_MOSI GPIO_Pin_15
#define SPI_PIN_MISO GPIO_Pin_14
#define SPI_PIN_CS GPIO_Pin_12
// 初始化SPI接口
void SPI_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
SPI_InitTypeDef SPI_InitStructure;
// 使能GPIO和SPI时钟
RCC_AHB1PeriphClockCmd(SPI_GPIO_CLK, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1, ENABLE);
// 配置SPI引脚
GPIO_InitStructure.GPIO_Pin = SPI_PIN_CLK | SPI_PIN_MISO | SPI_PIN_MOSI;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(SPI_GPIO_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = SPI_PIN_CS;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(SPI_GPIO_PORT, &GPIO_InitStructure);
// 将SPI引脚映射到SPI功能
GPIO_PinAFConfig(SPI_GPIO_PORT, GPIO_PinSource13, GPIO_AF_SPI1);
GPIO_PinAFConfig(SPI_GPIO_PORT, GPIO_PinSource14, GPIO_AF_SPI1);
GPIO_PinAFConfig(SPI_GPIO_PORT, GPIO_PinSource15, GPIO_AF_SPI1);
// 配置SPI接口
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_High;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_2Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_256;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStructure.SPI_CRCPolynomial = 7;
SPI_Init(SPI1, &SPI_InitStructure);
// 使能SPI接口
SPI_Cmd(SPI1, ENABLE);
}
// SPI发送一个字节
void SPI_SendByte(uint8_t byte)
{
// 等待SPI发送缓冲区为空
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
// 发送一个字节
SPI_I2S_SendData(SPI1, byte);
// 等待SPI接收缓冲区非空
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_RXNE) == RESET);
// 读取一个字节
SPI_I2S_ReceiveData(SPI1);
}
// 主函数
int main(void)
{
// 初始化SPI接口
SPI_Init();
// 无限循环
while (1)
{
// 发送一个字节
SPI_SendByte(0xAA);
// 延时一段时间
for (int i = 0; i < 1000000; i++);
}
}
```
注意,这里的代码仅仅是演示了如何使用STM32的SPI接口进行IO模拟,实际使用时需要根据具体的需求进行修改。同时,需要注意在使用SPI接口时要正确配置SPI的时钟极性、时钟相位等参数,否则可能会出现通信失败的问题。
阅读全文
相关推荐
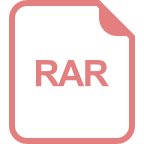
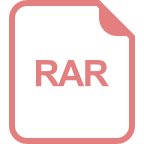
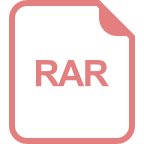
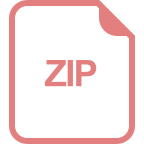
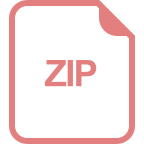
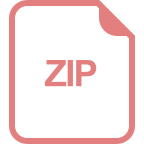
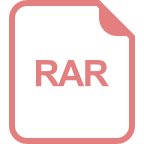
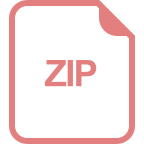
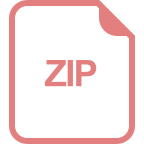
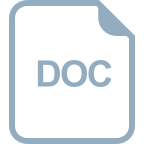
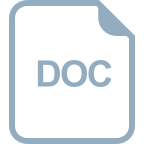
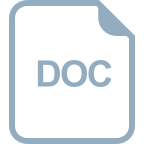
