Java语言编写程序演示多态的应用,要求:至少有1个抽象类和2个子类猫和狗。程序中要实现: (1)对象的向上转型和向下转型; (2)使用instanceof关键字判断对象所属类型; (3)方法要调用,程序要有输出结果。
时间: 2024-12-24 11:22:45 浏览: 8
在Java中,我们可以创建一个抽象类`Animal`作为多态的基础,并有两个具体的子类`Cat`和`Dog`来展示多态的应用。以下是简单的示例:
```java
// 抽象类 Animal 定义通用的行为
abstract class Animal {
abstract void makeSound(); // 抽象方法,子类需覆盖
// 上下转型示例
public void animalSound() {
System.out.println("This is an Animal.");
}
// 使用 instanceof 判断对象类型
public boolean isInstanceOf(Class<?> clazz) {
return this.getClass().isInstance(clazz);
}
}
// 子类 Cat 实现 Animal 的抽象方法
class Cat extends Animal {
@Override
void makeSound() {
System.out.println("Meow!");
}
}
// 子类 Dog 实现 Animal 的抽象方法
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Woof!");
}
}
public class Main {
public static void main(String[] args) {
// 创建 Animal 类型的对象数组
Animal[] animals = new Animal[2];
animals[0] = new Cat();
animals[1] = new Dog();
// 向上转型 - 动物声
for (Animal animal : animals) {
animal.animalSound(); // 输出各自的声音
}
// 下向转型 - 检查并打印动物类型
for (Animal animal : animals) {
if (animal instanceof Cat) {
System.out.println("This object is a " + animal.getClass().getSimpleName() + ".");
} else if (animal instanceof Dog) {
System.out.println("This object is a " + animal.getClass().getSimpleName() + ".");
}
}
}
}
```
运行此程序,你会看到输出类似这样的结果:
```
Meow!
This object is a Cat.
Woof!
This object is a Dog.
```
这个例子展示了如何通过向上转型调用通用方法,以及通过向下转型检查和打印对象的具体类型。
阅读全文
相关推荐

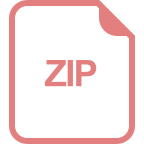
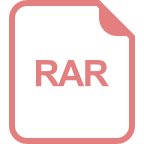
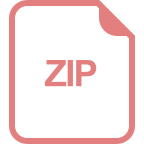
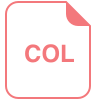
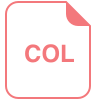
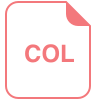
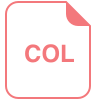
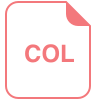
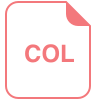
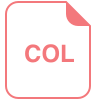
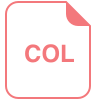
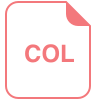
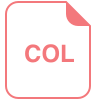
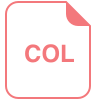
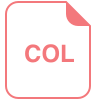
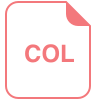
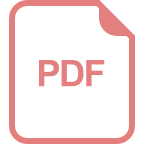
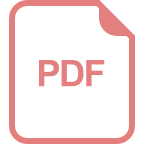