顺序表(1)建立有4个元素的线性表L={2,3,4,5} 插入:在L={2,3,45}的元素4与5之间插入一个元素9,实现插入的基本操作;(3)在L={2,3,49,5}中删除指定位置(i=3 )上的元素c语言
时间: 2024-09-27 19:07:11 浏览: 111
在C语言中,我们可以创建一个动态顺序表结构来存储整数,并实现插入和删除操作。首先,我们需要定义一个结构体表示每个元素和一个数组来保存顺序表数据。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct List {
Node* head;
int size;
} List;
// 插入函数,在给定位置插入新元素
void insert(List* list, int value, int position) {
if (list == NULL || position < 0 || position > list->size + 1) {
printf("Invalid operation.\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = list->head;
if (position == 0) {
list->head = newNode;
} else {
Node* current = list->head;
for (int i = 0; i < position - 1; i++) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
list->size++;
}
// 删除函数,删除指定索引位置的元素
void delete(List* list, int index) {
if (list == NULL || index < 0 || index >= list->size) {
printf("Invalid index.\n");
return;
}
if (index == 0) {
list->head = list->head->next;
} else {
Node* current = list->head;
for (int i = 0; i < index - 1; i++) {
current = current->next;
}
current->next = current->next->next;
}
free(list->head); // 如果删除的是第一个节点,记得释放内存
list->size--;
}
// 示例:建立初始列表
List createList(int arr[], int length) {
List list;
list.head = NULL;
list.size = 0;
for (int i = 0; i < length; i++) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = arr[i];
node->next = NULL;
if (list.head == NULL) {
list.head = node;
} else {
Node* last = list.head;
while (last->next != NULL) {
last = last->next;
}
last->next = node;
}
list.size++;
}
return list;
}
int main() {
int arr[] = {2, 3, 4, 5};
List list = createList(arr, sizeof(arr) / sizeof(arr[0]));
// 插入示例
insert(&list, 9, 2);
printf("After insertion: ");
printList(list);
// 删除示例
delete(&list, 3);
printf("\nAfter deletion at index 3: ");
printList(list);
return 0;
}
// 打印列表辅助函数
void printList(const List& list) {
Node* current = list.head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
在这个例子中,我们首先创建了一个包含{2, 3, 4, 5}的列表,然后分别演示了如何在元素4和5之间插入元素9,以及在第3个位置(索引3)删除元素。注意`delete`函数处理了特殊情况,即删除第一个节点时需要释放头指针所占用的内存。最后展示了修改后的列表。
阅读全文
相关推荐
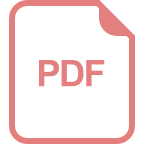
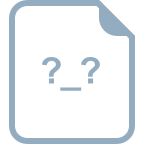
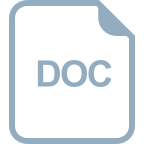















