建立一个链式线性表L=(1,2,3,4,5,6),实现对顺序表的建立插入和删除,用c++语言进行实现
时间: 2024-11-07 13:13:37 浏览: 12
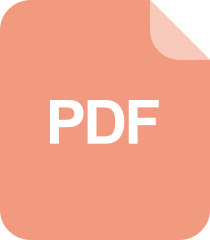
2-2基于链式存储的线性表的表示与实现.pdf
在C++中,我们可以创建一个简单的链表结构来表示顺序线性表,并提供插入和删除操作。这里是一个基础的单向链表节点和链表类的示例:
```cpp
#include <iostream>
// 链表节点定义
struct Node {
int data;
Node* next; // 指针指向下一个节点
Node(int value) : data(value), next(nullptr) {} // 构造函数
};
// 链表类
class LinkedList {
private:
Node* head; // 链表头指针
public:
LinkedList() : head(nullptr) {}
// 插入元素到链表尾部
void insert(int value) {
if (!head) {
head = new Node(value);
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new Node(value);
}
}
// 删除指定值的第一个节点
void deleteElement(int value) {
if (head == nullptr || head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
Node* temp = current->next;
current->next = current->next->next;
delete temp;
}
}
// 打印链表内容
void printList() {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
};
int main() {
LinkedList list;
list.insert(1);
list.insert(2);
list.insert(3);
list.insert(4);
list.insert(5);
list.insert(6);
list.printList(); // 输出: 1 2 3 4 5 6
list.deleteElement(3);
list.deleteElement(6);
list.printList(); // 输出: 1 2 4 5
return 0;
}
```
这个程序首先创建了一个链表`list`,然后依次插入数字1到6,接着删除值为3和6的节点,并打印出更新后的链表内容。
阅读全文
相关推荐
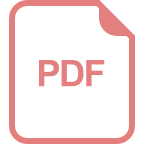
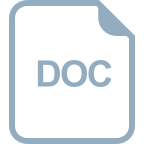


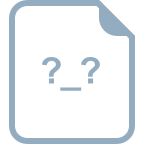
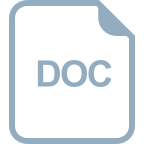
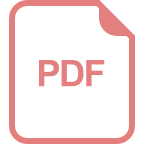
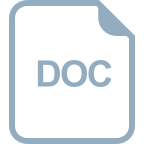
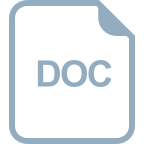
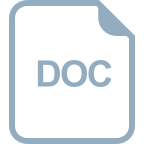
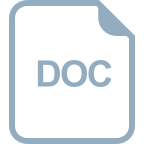
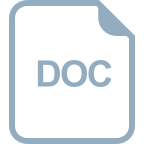
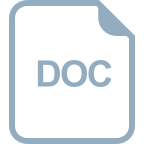
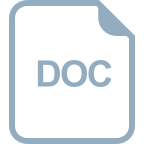
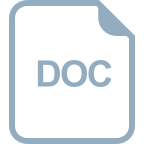
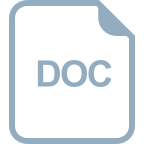
