NameError: name 'y' is not defined
时间: 2023-09-09 07:06:27 浏览: 262
抱歉,这是因为代码中没有定义变量y,需要在代码中定义y的取值范围。下面是更新后的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义x和y的取值范围
x = np.arange(-50, 50, 0.1)
y = np.arange(-50, 50, 0.1)
# 计算出y1和y2的值
y1 = -x + 2 * (0.5 * x + 0.5 * y + 45) - 90
y2 = -4 * x - 10 * (0.5 * x + 0.5 * y + 45) + 490
# 绘制直线
plt.plot(x, y1, label='Line 1')
plt.plot(x, y2, label='Line 2')
# 添加标签和标题
plt.xlabel('x')
plt.ylabel('y')
plt.title('Two Lines')
# 显示图例
plt.legend()
# 显示图形
plt.show()
```
运行更新后的代码,即可得到绘制出的两条直线图像。
相关问题
NameError: name 'AIFI' is not defined
NameError: name 'AIFI' is not defined 是Python中的一个错误提示,表示使用了一个未定义的变量或函数名。这通常是由于以下几种情况引起的:
1. 拼写错误:检查变量或函数名的拼写是否正确。Python是区分大小写的,所以确保大小写匹配。
2. 变量未赋值:如果尝试使用一个未赋值的变量,就会出现NameError。在使用变量之前,确保已经为其赋值。
3. 变量作用域问题:如果变量在当前作用域中未定义,但在其他作用域中定义了,也会出现NameError。确保变量在使用之前在当前作用域中定义。
4. 导入模块问题:如果尝试使用未导入的模块或未导入模块中的函数或变量,也会出现NameError。确保已经正确导入所需的模块。
下面是一个示例代码,演示了NameError的情况:
```python
# 拼写错误
print(AIFI) # NameError: name 'AIFI' is not defined
# 变量未赋值
x = 10
print(y) # NameError: name 'y' is not defined
# 变量作用域问题
def test():
print(x) # NameError: name 'x' is not defined
test()
# 导入模块问题
import math
print(math.pi) # 正确,输出:3.141592653589793
print(math.e) # 正确,输出:2.718281828459045
print(math.sin(0)) # 正确,输出:0.0
print(sin(0)) # NameError: name 'sin' is not defined
```
NameError: name 'correlation' is not defined
`NameError: name 'correlation' is not defined` 这个错误通常发生在尝试访问未定义的变量 `correlation` 时。在之前的示例中,如果你忘记在计算相关性之前定义它,就会遇到这个问题。
确保你在计算完相关系数之后才引用它。这里是完整且修正过的代码片段:
```python
import pandas as pd
import numpy as np
from scipy.stats import pearsonr
import matplotlib.pyplot as plt
# 读取 Excel 文件
df = pd.read_excel('data.xlsx', sheet_name='Sheet1')
# 筛选数据
df_filtered = df[df['KC1'] == '高等数学']
# 确保有数据才能继续
if not df_filtered.empty:
# 提取需要的列数据
cj1_data = df_filtered['CJ1'].values
ywk2_data = df_filtered['YWK2'].values
# 计算皮尔逊相关系数
correlation, _ = pearsonr(cj1_data, ywk2_data)
print(f"CJ1和YWK2列的相关性为:{correlation}")
# 可视化相关性
if not correlation is None:
plt.scatter(cj1_data, ywk2_data)
plt.xlabel('CJ1')
plt.ylabel('YWK2')
plt.title(f"CJ1与YWK2的相关性:{correlation:.2f}")
plt.show()
else:
print("筛选后的数据为空,无法计算相关性")
```
在这个修正版中,我们在计算相关性之后才赋值给 `correlation` 变量,避免了 NameError 的发生。
阅读全文
相关推荐
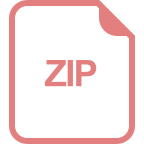
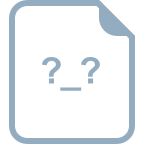
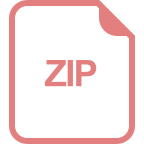













