任意给定的直线和圆的方程,求它们公切圆的方程c语言
时间: 2024-02-27 17:56:35 浏览: 45
假设直线的方程为 ax + by + c = 0,圆的方程为 (x - p)² + (y - q)² = r²。
公切圆有两种情况:内切和外切。内切时,直线和圆在同一侧,外切时在相反侧。我们需要分别求解这两种情况。
内切圆的方程:
1. 计算直线与圆心的距离 d = |ap + bq + c| / sqrt(a² + b²)。
2. 圆半径 r 和距离 d 一致,即 r = d。
3. 圆心坐标为圆心到直线距离的向量(向量长度为 r),即 x = p + (br / sqrt(a² + b²)),y = q - (ar / sqrt(a² + b²))。
4. 将圆心和半径代入圆的标准方程即可得到内切圆的方程。
C 语言代码:
```c
#include <stdio.h>
#include <math.h>
int main() {
double a, b, c, p, q, r, d, x, y;
printf("Enter the equation of the line ax + by + c = 0: ");
scanf("%lf%lf%lf", &a, &b, &c);
printf("Enter the equation of the circle (x - p)^2 + (y - q)^2 = r^2: ");
scanf("%lf%lf%lf", &p, &q, &r);
// 计算直线与圆心的距离
d = fabs(a * p + b * q + c) / sqrt(a * a + b * b);
// 计算圆心坐标
x = p + b * r / sqrt(a * a + b * b);
y = q - a * r / sqrt(a * a + b * b);
// 判断内切还是外切
if (d < r) {
printf("The circle is inside the line.\n");
} else if (d == r) {
printf("The circle is tangent to the line.\n");
} else {
printf("The circle is outside the line.\n");
}
// 输出内切圆的方程
printf("The equation of the inscribed circle is: (x - %.2lf)^2 + (y - %.2lf)^2 = %.2lf^2\n", x, y, r);
return 0;
}
```
外切圆的方程:
1. 计算直线与圆心的距离 d = |ap + bq + c| / sqrt(a² + b²)。
2. 圆半径 r 和距离 d 一致,即 r = d。
3. 圆心坐标为圆心到直线距离的向量的反向(向量长度为 r),即 x = p - (br / sqrt(a² + b²)),y = q + (ar / sqrt(a² + b²))。
4. 将圆心和半径代入圆的标准方程即可得到外切圆的方程。
C 语言代码:
```c
#include <stdio.h>
#include <math.h>
int main() {
double a, b, c, p, q, r, d, x, y;
printf("Enter the equation of the line ax + by + c = 0: ");
scanf("%lf%lf%lf", &a, &b, &c);
printf("Enter the equation of the circle (x - p)^2 + (y - q)^2 = r^2: ");
scanf("%lf%lf%lf", &p, &q, &r);
// 计算直线与圆心的距离
d = fabs(a * p + b * q + c) / sqrt(a * a + b * b);
// 计算圆心坐标
x = p - b * r / sqrt(a * a + b * b);
y = q + a * r / sqrt(a * a + b * b);
// 判断内切还是外切
if (d < r) {
printf("The circle is inside the line.\n");
} else if (d == r) {
printf("The circle is tangent to the line.\n");
} else {
printf("The circle is outside the line.\n");
}
// 输出外切圆的方程
printf("The equation of the circumscribed circle is: (x - %.2lf)^2 + (y - %.2lf)^2 = %.2lf^2\n", x, y, r);
return 0;
}
```
阅读全文
相关推荐
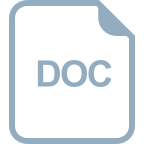
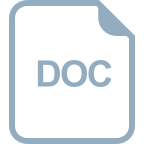
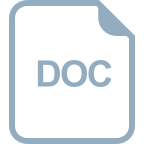




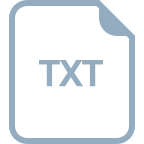
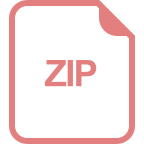
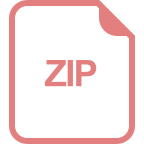
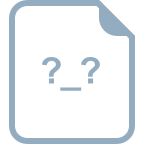
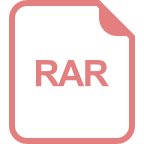






