用c语言建立一个4层完全二叉树,使其具有建立排序二叉树插入结点和删除节点的功能
时间: 2023-06-18 20:05:40 浏览: 156
以下是一个基于C语言的4层完全二叉树代码示例,具有插入和删除节点的功能。这个树是一个排序二叉树,每个节点都包含一个整数值。
```
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结构
typedef struct node {
int value;
struct node *left;
struct node *right;
} Node;
// 创建一个新节点
Node *newNode(int value) {
Node *node = (Node *) malloc(sizeof(Node));
node->value = value;
node->left = NULL;
node->right = NULL;
return node;
}
// 向树中插入一个节点
Node *insert(Node *root, int value) {
if (root == NULL) {
return newNode(value);
} else if (value < root->value) {
root->left = insert(root->left, value);
} else if (value > root->value) {
root->right = insert(root->right, value);
}
return root;
}
// 删除树中的一个节点
Node *delete(Node *root, int value) {
if (root == NULL) {
return root;
} else if (value < root->value) {
root->left = delete(root->left, value);
} else if (value > root->value) {
root->right = delete(root->right, value);
} else {
// 找到要删除的节点
if (root->left == NULL) {
Node *temp = root->right;
free(root);
return temp;
} else if (root->right == NULL) {
Node *temp = root->left;
free(root);
return temp;
}
// 找到右子树中的最小值
Node *temp = root->right;
while (temp && temp->left != NULL) {
temp = temp->left;
}
// 将右子树中的最小值替换到当前节点
root->value = temp->value;
root->right = delete(root->right, temp->value);
}
return root;
}
// 打印树中的所有值
void printTree(Node *root) {
if (root != NULL) {
printTree(root->left);
printf("%d ", root->value);
printTree(root->right);
}
}
int main() {
Node *root = NULL;
root = insert(root, 5);
insert(root, 3);
insert(root, 1);
insert(root, 4);
insert(root, 7);
insert(root, 6);
insert(root, 8);
printf("原始树:");
printTree(root);
printf("\n");
root = delete(root, 5);
printf("删除节点后的树:");
printTree(root);
printf("\n");
return 0;
}
```
在这个代码示例中,我们使用递归算法来插入和删除节点。插入节点的方法从根节点开始,如果节点不存在,则创建一个新节点。如果节点已经存在,则继续递归到左子树或右子树中,直到找到一个空的位置。删除节点的方法也使用递归算法,在找到要删除的节点后,根据其子节点的情况进行相应的操作。
需要注意的是,在删除节点时,我们需要考虑到树的平衡性,以避免出现严重的不平衡情况。这个示例代码中没有实现平衡性,需要根据实际情况进行改进。
阅读全文
相关推荐
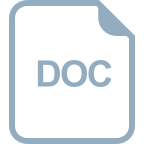
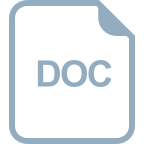
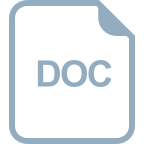















