用c语言编写代码:向一棵二叉树,插入一个结点的算法
时间: 2023-05-25 21:01:28 浏览: 74
下面是用C语言实现向一棵二叉树插入一个节点的算法:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int val; // 节点的值
struct node* left; // 左子树
struct node* right; // 右子树
};
struct node* create_new_node(int val) {
struct node* new_node = (struct node*) malloc(sizeof(struct node));
new_node->val = val;
new_node->left = NULL;
new_node->right = NULL;
return new_node;
}
// 插入节点的函数
struct node* insert_node(struct node* root, int val) {
if (root == NULL) {
// 如果树为空,则直接创建一个新节点作为根节点
struct node* new_node = create_new_node(val);
return new_node;
} else {
// 否则,插入节点
if (val <= root->val) {
root->left = insert_node(root->left, val);
} else {
root->right = insert_node(root->right, val);
}
return root;
}
}
int main() {
struct node* root = create_new_node(5); // 创建根节点
insert_node(root, 3); // 插入节点3
insert_node(root, 8); // 插入节点8
insert_node(root, 4); // 插入节点4
insert_node(root, 6); // 插入节点6
insert_node(root, 2); // 插入节点2
printf("Inorder traversal of the binary search tree is:\n");
inorder_traversal(root); // 中序遍历二叉树
return 0;
}
```
在上面的代码中,我们首先定义了一个结构体`node`,表示树的节点。每个节点包含了一个值和指向左右子树的指针。
`create_new_node()`函数用于创建一个新的节点,并返回该节点的指针。
`insert_node()`函数用于向二叉树中插入一个节点。如果树为空,则直接创建一个新节点作为根节点;否则,根据节点值的大小关系,将节点插入到左子树或右子树中。
最后,在`main()`函数中,我们创建根节点,插入若干个节点,然后使用`inorder_traversal()`函数中序遍历二叉树,输出所有节点的值。
相关推荐
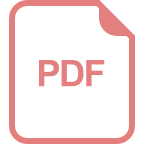
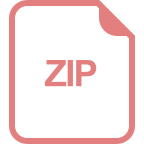














