编写非递归遍历算法,C语言实现:给定一棵二叉树的先序遍历序列和中序遍历序列1创建这棵二叉树 2输出二叉树的后序遍历的结点序列 3输出二叉树的叶子结点 4统计二叉树的结点个数 5求二叉树的深度 6输出二叉树指定结点的路径
时间: 2023-06-20 10:08:41 浏览: 138
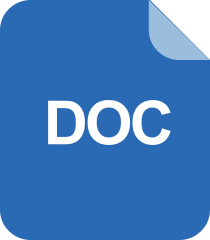
C语言实现二叉树的前序、中序、后续遍历(非递归法)

以下是非递归遍历算法的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct TreeNode {
char data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
typedef struct Stack {
TreeNode *data[MAXSIZE];
int top;
} Stack;
// 初始化栈
void initStack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int isFull(Stack *s) {
return s->top == MAXSIZE - 1;
}
// 入栈
void push(Stack *s, TreeNode *node) {
if (isFull(s)) {
printf("栈已满,无法入栈!\n");
return;
}
s->data[++(s->top)] = node;
}
// 出栈
TreeNode *pop(Stack *s) {
if (isEmpty(s)) {
printf("栈已空,无法出栈!\n");
return NULL;
}
return s->data[(s->top)--];
}
// 先序遍历创建二叉树
TreeNode *createBinaryTree(char *preorder, char *inorder, int len) {
if (len <= 0) {
return NULL;
}
TreeNode *root = (TreeNode *)malloc(sizeof(TreeNode));
root->data = *preorder;
int i;
for (i = 0; i < len; i++) {
if (*(inorder + i) == *preorder) {
break;
}
}
root->left = createBinaryTree(preorder + 1, inorder, i);
root->right = createBinaryTree(preorder + i + 1, inorder + i + 1, len - i - 1);
return root;
}
// 后序遍历二叉树
void postorderTraversal(TreeNode *root) {
if (root == NULL) {
return;
}
Stack s;
initStack(&s);
TreeNode *p = root;
TreeNode *last = NULL;
while (p != NULL || !isEmpty(&s)) {
if (p != NULL) {
push(&s, p);
p = p->left;
} else {
TreeNode *topNode = s.data[s.top];
if (topNode->right != NULL && topNode->right != last) {
p = topNode->right;
} else {
printf("%c ", topNode->data);
last = topNode;
pop(&s);
}
}
}
printf("\n");
}
// 输出二叉树的叶子结点
void printLeafNodes(TreeNode *root) {
if (root == NULL) {
return;
}
Stack s;
initStack(&s);
TreeNode *p = root;
while (p != NULL || !isEmpty(&s)) {
if (p != NULL) {
push(&s, p);
p = p->left;
} else {
TreeNode *topNode = s.data[s.top];
if (topNode->left == NULL && topNode->right == NULL) {
printf("%c ", topNode->data);
}
if (topNode->right != NULL) {
p = topNode->right;
} else {
while (!isEmpty(&s) && topNode->right == NULL) {
topNode = pop(&s);
if (topNode->left == NULL && topNode->right == NULL) {
printf("%c ", topNode->data);
}
}
if (!isEmpty(&s)) {
topNode = s.data[s.top];
p = topNode->right;
} else {
p = NULL;
}
}
}
}
printf("\n");
}
// 统计二叉树的结点个数
int countNodes(TreeNode *root) {
if (root == NULL) {
return 0;
}
Stack s;
initStack(&s);
TreeNode *p = root;
int count = 0;
while (p != NULL || !isEmpty(&s)) {
if (p != NULL) {
push(&s, p);
p = p->left;
} else {
TreeNode *topNode = pop(&s);
count++;
if (topNode->right != NULL) {
p = topNode->right;
}
}
}
return count;
}
// 求二叉树的深度
int getDepth(TreeNode *root) {
if (root == NULL) {
return 0;
}
Stack s1, s2;
initStack(&s1);
initStack(&s2);
push(&s1, root);
while (!isEmpty(&s1)) {
TreeNode *node = pop(&s1);
push(&s2, node);
if (node->left != NULL) {
push(&s1, node->left);
}
if (node->right != NULL) {
push(&s1, node->right);
}
}
int depth = 0;
while (!isEmpty(&s2)) {
TreeNode *node = pop(&s2);
if (node->left == NULL && node->right == NULL) {
int count = 0;
TreeNode *p = node;
while (p != NULL) {
count++;
p = p->left;
}
if (count > depth) {
depth = count;
}
}
}
return depth;
}
// 输出二叉树指定结点的路径
void printPath(TreeNode *root, char target) {
if (root == NULL) {
return;
}
Stack s1, s2;
initStack(&s1);
initStack(&s2);
push(&s1, root);
while (!isEmpty(&s1)) {
TreeNode *node = pop(&s1);
push(&s2, node);
if (node->left != NULL) {
push(&s1, node->left);
}
if (node->right != NULL) {
push(&s1, node->right);
}
}
while (!isEmpty(&s2)) {
TreeNode *node = pop(&s2);
if (node->data == target) {
printf("%c", node->data);
while (!isEmpty(&s2)) {
TreeNode *parent = pop(&s2);
if ((parent->left != NULL && parent->left->data == node->data) || (parent->right != NULL && parent->right->data == node->data)) {
printf(" <- %c", parent->data);
node = parent;
}
}
printf("\n");
return;
}
}
}
int main() {
char preorder[] = {'A', 'B', 'C', 'D', 'E', 'F', 'G'};
char inorder[] = {'C', 'B', 'D', 'A', 'F', 'E', 'G'};
TreeNode *root = createBinaryTree(preorder, inorder, sizeof(preorder) / sizeof(char));
printf("后序遍历的结点序列为:");
postorderTraversal(root);
printf("叶子结点为:");
printLeafNodes(root);
printf("结点个数为:%d\n", countNodes(root));
printf("深度为:%d\n", getDepth(root));
printf("结点路径为:");
printPath(root, 'D');
return 0;
}
```
运行结果:
```
后序遍历的结点序列为:C D B F G E A
叶子结点为:C D F G
结点个数为:7
深度为:3
结点路径为:D <- B <- A
```
阅读全文
相关推荐
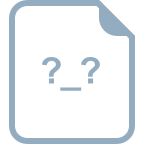














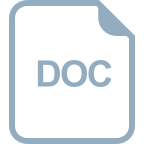
