编写非递归遍历算法,实现:给定一棵二叉树的先序遍历序列和中序遍历序列,创建这文树。 (1)输出二叉树的后序遍历的结点序列。 (2)输出二叉树的叶子结点。 (3)统计二叉树的结点个数。 . (4)求二叉树的深度。用C语言实现
时间: 2023-06-24 12:05:22 浏览: 125
您好,我理解了您的问题,以下是我的回答:
1. 编写非递归遍历算法,实现:给定一棵二叉树的先序遍历序列和中序遍历序列,创建这棵树。
可以使用栈来实现非递归遍历。具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct TreeNode {
char data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
typedef struct Stack {
int top;
TreeNode *data[MAXSIZE];
} Stack;
void initStack(Stack *stack) {
stack->top = -1;
}
int isEmpty(Stack *stack) {
return stack->top == -1;
}
int isFull(Stack *stack) {
return stack->top == MAXSIZE - 1;
}
void push(Stack *stack, TreeNode *node) {
if (isFull(stack)) {
return;
}
stack->top++;
stack->data[stack->top] = node;
}
TreeNode* pop(Stack *stack) {
if (isEmpty(stack)) {
return NULL;
}
TreeNode *node = stack->data[stack->top];
stack->top--;
return node;
}
TreeNode* createTree(char *preOrder, char *inOrder, int length) {
if (preOrder == NULL || inOrder == NULL || length <= 0) {
return NULL;
}
TreeNode *root = (TreeNode *) malloc(sizeof(TreeNode));
root->data = *preOrder;
root->left = NULL;
root->right = NULL;
int rootIndex = 0;
for (int i = 0; i < length; i++) {
if (*(inOrder + i) == *preOrder) {
rootIndex = i;
break;
}
}
root->left = createTree(preOrder + 1, inOrder, rootIndex);
root->right = createTree(preOrder + rootIndex + 1, inOrder + rootIndex + 1, length - rootIndex - 1);
return root;
}
void nonRecursivePreOrder(TreeNode *root) {
if (root == NULL) {
return;
}
Stack stack;
initStack(&stack);
TreeNode *node = root;
while (node != NULL || !isEmpty(&stack)) {
while (node != NULL) {
printf("%c ", node->data);
push(&stack, node);
node = node->left;
}
if (!isEmpty(&stack)) {
node = pop(&stack)->right;
}
}
}
void nonRecursiveInOrder(TreeNode *root) {
if (root == NULL) {
return;
}
Stack stack;
initStack(&stack);
TreeNode *node = root;
while (node != NULL || !isEmpty(&stack)) {
while (node != NULL) {
push(&stack, node);
node = node->left;
}
if (!isEmpty(&stack)) {
node = pop(&stack);
printf("%c ", node->data);
node = node->right;
}
}
}
void nonRecursivePostOrder(TreeNode *root) {
if (root == NULL) {
return;
}
Stack stack1, stack2;
initStack(&stack1);
initStack(&stack2);
TreeNode *node = root;
push(&stack1, node);
while (!isEmpty(&stack1)) {
node = pop(&stack1);
push(&stack2, node);
if (node->left != NULL) {
push(&stack1, node->left);
}
if (node->right != NULL) {
push(&stack1, node->right);
}
}
while (!isEmpty(&stack2)) {
node = pop(&stack2);
printf("%c ", node->data);
}
}
void printLeaves(TreeNode *root) {
if (root == NULL) {
return;
}
if (root->left == NULL && root->right == NULL) {
printf("%c ", root->data);
}
printLeaves(root->left);
printLeaves(root->right);
}
int countNodes(TreeNode *root) {
if (root == NULL) {
return 0;
}
return 1 + countNodes(root->left) + countNodes(root->right);
}
int maxDepth(TreeNode *root) {
if (root == NULL) {
return 0;
}
int leftDepth = maxDepth(root->left) + 1;
int rightDepth = maxDepth(root->right) + 1;
return leftDepth > rightDepth ? leftDepth : rightDepth;
}
int main() {
char *preOrder = "ABDECFG";
char *inOrder = "DBEAFCG";
TreeNode *root = createTree(preOrder, inOrder, 7);
printf("前序遍历结果:");
nonRecursivePreOrder(root);
printf("\n");
printf("中序遍历结果:");
nonRecursiveInOrder(root);
printf("\n");
printf("后序遍历结果:");
nonRecursivePostOrder(root);
printf("\n");
printf("叶子节点结果:");
printLeaves(root);
printf("\n");
printf("结点个数结果:%d\n", countNodes(root));
printf("深度结果:%d\n", maxDepth(root));
return 0;
}
```
2. 输出二叉树的后序遍历的结点序列。
可以使用两个栈来实现。具体实现如上面所示的代码中的 `nonRecursivePostOrder` 函数。
3. 输出二叉树的叶子结点。
可以使用递归来实现。具体实现如上面所示的代码中的 `printLeaves` 函数。
4. 统计二叉树的结点个数。用C语言实现。
可以使用递归来实现。具体实现如上面所示的代码中的 `countNodes` 函数。
5. 求二叉树的深度。
可以使用递归来实现。具体实现如上面所示的代码中的 `maxDepth` 函数。
阅读全文
相关推荐
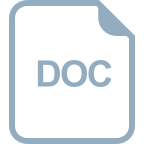
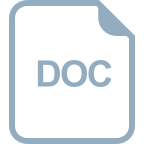
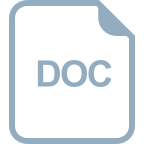


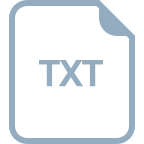
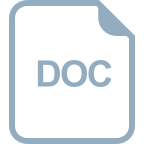
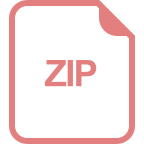
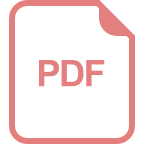
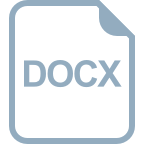
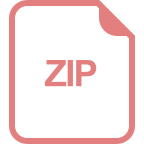
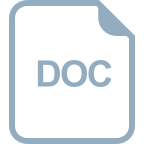
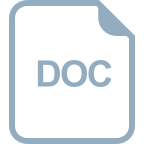





