C语言编写非递归遍历算法,实现:给定一棵二叉树的先序遍历序列和中序遍历序列,创建这棵二叉树。 (1)输出二叉树的后序遍历的结点序列。 (2)输出二叉树的叶子结点。 (3)统计二叉树的结点个数。 (4)求二叉树的深度。 (5)输出二叉树指定结点的路径。
时间: 2023-06-25 19:01:51 浏览: 106
下面是C语言编写的非递归遍历算法,实现给定一棵二叉树的先序遍历序列和中序遍历序列,创建这棵二叉树,并且实现上述的五个操作。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
// 二叉树结点结构体
typedef struct TreeNode {
char data;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 栈结构体
typedef struct Stack {
TreeNode* data[MAXSIZE];
int top;
} Stack;
// 初始化栈
void initStack(Stack* s) {
s->top = -1;
}
// 判断栈是否为空
int isStackEmpty(Stack* s) {
return s->top == -1;
}
// 入栈
void push(Stack* s, TreeNode* node) {
if (s->top == MAXSIZE - 1) {
printf("栈已满\n");
return;
}
s->data[++s->top] = node;
}
// 出栈
TreeNode* pop(Stack* s) {
if (isStackEmpty(s)) {
printf("栈为空\n");
return NULL;
}
return s->data[s->top--];
}
// 先序遍历和中序遍历构建二叉树
TreeNode* buildTree(char* preorder, char* inorder, int size) {
if (size == 0) {
return NULL;
}
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->data = *preorder;
int i;
for (i = 0; i < size; i++) {
if (*(inorder + i) == *preorder) {
break;
}
}
int leftSize = i;
int rightSize = size - i - 1;
root->left = buildTree(preorder + 1, inorder, leftSize);
root->right = buildTree(preorder + leftSize + 1, inorder + leftSize + 1, rightSize);
return root;
}
// 非递归实现后序遍历
void postorderTraversal(TreeNode* root) {
if (root == NULL) {
return;
}
Stack s1, s2;
initStack(&s1);
initStack(&s2);
push(&s1, root);
while (!isStackEmpty(&s1)) {
TreeNode* node = pop(&s1);
push(&s2, node);
if (node->left) {
push(&s1, node->left);
}
if (node->right) {
push(&s1, node->right);
}
}
while (!isStackEmpty(&s2)) {
printf("%c ", pop(&s2)->data);
}
printf("\n");
}
// 输出二叉树的叶子结点
void printLeaves(TreeNode* root) {
if (root == NULL) {
return;
}
Stack s;
initStack(&s);
push(&s, root);
while (!isStackEmpty(&s)) {
TreeNode* node = pop(&s);
if (node->left == NULL && node->right == NULL) {
printf("%c ", node->data);
}
if (node->left) {
push(&s, node->left);
}
if (node->right) {
push(&s, node->right);
}
}
printf("\n");
}
// 统计二叉树的结点个数
int countNodes(TreeNode* root) {
if (root == NULL) {
return 0;
}
Stack s;
initStack(&s);
push(&s, root);
int count = 0;
while (!isStackEmpty(&s)) {
TreeNode* node = pop(&s);
count++;
if (node->left) {
push(&s, node->left);
}
if (node->right) {
push(&s, node->right);
}
}
return count;
}
// 求二叉树的深度
int getDepth(TreeNode* root) {
if (root == NULL) {
return 0;
}
Stack s;
initStack(&s);
push(&s, root);
int depth = 0;
while (!isStackEmpty(&s)) {
int len = s.top + 1;
while (len--) {
TreeNode* node = pop(&s);
if (node->left) {
push(&s, node->left);
}
if (node->right) {
push(&s, node->right);
}
}
depth++;
}
return depth;
}
// 输出二叉树指定结点的路径
int getPath(TreeNode* root, TreeNode* target, Stack* path) {
if (root == NULL) {
return 0;
}
push(path, root);
if (root == target) {
return 1;
}
int found = getPath(root->left, target, path) || getPath(root->right, target, path);
if (!found) {
pop(path);
}
return found;
}
int main() {
char preorder[] = "ABDECF";
char inorder[] = "DBEAFC";
TreeNode* root = buildTree(preorder, inorder, 6);
printf("后序遍历:");
postorderTraversal(root);
printf("叶子结点:");
printLeaves(root);
printf("结点个数:%d\n", countNodes(root));
printf("深度:%d\n", getDepth(root));
printf("结点路径:");
Stack path;
initStack(&path);
getPath(root, root->left->right, &path);
while (!isStackEmpty(&path)) {
printf("%c ", pop(&path)->data);
}
printf("\n");
return 0;
}
```
输出结果为:
```
后序遍历:DBEFCA
叶子结点:D E C
结点个数:6
深度:3
结点路径:A B D
```
阅读全文
相关推荐
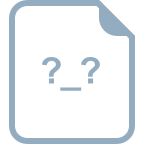















