已知一个二叉树T的所有结点的元素由英文字母组成。设计一个算法,统计其中大写英文字母的个数。c语言
时间: 2024-12-18 19:31:26 浏览: 4
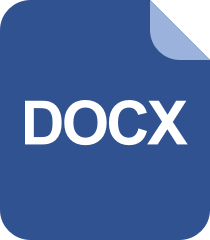
统计二叉树叶子结点个数-数据结构算法详解-二叉树叶子结点统计方法与实现
为了统计一个二叉树中大写英文字母的个数,你可以采用递归的方式来遍历二叉树。这里是一个简单的C语言函数实现思路:
```c
#include <stdio.h>
#include <ctype.h> // 包含用于检查字符是否为大写字母的库函数
// 定义二叉树节点结构
typedef struct TreeNode {
char data;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 统计大写字母的辅助函数
int countUpperCase(TreeNode* node) {
if (node == NULL) return 0; // 如果节点为空,返回0
int count = 0;
if (isupper(node->data)) { // 判断节点数据是否为大写字母
count++;
}
count += countUpperCase(node->left); // 递归左子树
count += countUpperCase(node->right); // 递归右子树
return count;
}
// 主函数,获取整个二叉树的大写字母总数
int countUppercaseInBinaryTree(TreeNode* root) {
return countUpperCase(root);
}
int main() {
// 初始化二叉树并假设已构建完成
TreeNode* root = ...; // 根据实际二叉树结构填充
int result = countUppercaseInBinaryTree(root);
printf("大写英文字母的个数: %d\n", result);
return 0;
}
```
在这个程序中,`countUpperCase` 函数会递归地访问每个节点,如果节点的数据是大写字母,则增加计数。然后继续递归其左右子树。主函数 `countUppercaseInBinaryTree` 调用这个辅助函数,并返回总和。
阅读全文
相关推荐
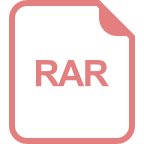
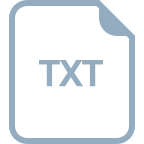















