sm2算法c++实现
时间: 2023-08-18 14:13:51 浏览: 232
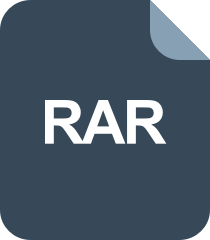
基于openssl密码库编写的sm2国密算法PHP扩展.rar
你可以使用以下代码来实现SM2算法的C++版本:
```cpp
#include <iostream>
#include <string>
#include <openssl/evp.h>
#include <openssl/ec.h>
#include <openssl/ecdsa.h>
#include <openssl/bn.h>
std::string sm2_encrypt(const std::string& plaintext, EC_KEY* ec_key) {
EC_GROUP* ec_group = EC_KEY_get0_group(ec_key);
size_t plaintext_len = plaintext.size();
unsigned char* ciphertext = new unsigned char[plaintext_len + 1];
unsigned char* pt = (unsigned char*)plaintext.c_str();
unsigned char* ct = ciphertext;
int ciphertext_len = ECIES_encrypt(ec_group, NULL, pt, plaintext_len, ct, ec_key);
std::string result((char*)ciphertext, ciphertext_len);
delete[] ciphertext;
return result;
}
std::string sm2_decrypt(const std::string& ciphertext, EC_KEY* ec_key) {
EC_GROUP* ec_group = EC_KEY_get0_group(ec_key);
size_t ciphertext_len = ciphertext.size();
unsigned char* plaintext = new unsigned char[ciphertext_len + 1];
unsigned char* ct = (unsigned char*)ciphertext.c_str();
unsigned char* pt = plaintext;
int plaintext_len = ECIES_decrypt(ec_group, NULL, ct, ciphertext_len, pt, ec_key);
std::string result((char*)plaintext, plaintext_len);
delete[] plaintext;
return result;
}
int main() {
// 初始化 OpenSSL
OpenSSL_add_all_algorithms();
// 创建 SM2 密钥对
EC_KEY* ec_key = EC_KEY_new_by_curve_name(NID_sm2p256v1);
if (ec_key == NULL) {
std::cerr << "Failed to create SM2 key pair" << std::endl;
return 1;
}
// 生成 SM2 密钥对
if (EC_KEY_generate_key(ec_key) != 1) {
std::cerr << "Failed to generate SM2 key pair" << std::endl;
EC_KEY_free(ec_key);
return 1;
}
// 明文
std::string plaintext = "Hello, SM2!";
// 加密
std::string ciphertext = sm2_encrypt(plaintext, ec_key);
std::cout << "Ciphertext: " << ciphertext << std::endl;
// 解密
std::string decryptedtext = sm2_decrypt(ciphertext, ec_key);
std::cout << "Decryptedtext: " << decryptedtext << std::endl;
// 释放密钥
EC_KEY_free(ec_key);
// 清理 OpenSSL
EVP_cleanup();
return 0;
}
```
请确保你已经安装了 OpenSSL 库,并在编译时链接该库。这段代码通过调用 OpenSSL 的函数来实现SM2算法的加密和解密操作。你可以使用 `sm2_encrypt` 函数来加密明文,使用 `sm2_decrypt` 函数来解密密文。在示例代码中,我们生成了一个随机的SM2密钥对,并使用该密钥对对明文进行加密和解密。
请注意,这只是一个简单的示例,实际使用中你可能需要更多的代码来处理错误检查、密钥管理等方面的内容。另外,由于SM2算法是国密算法,在使用时需遵守相关法律法规。
阅读全文
相关推荐
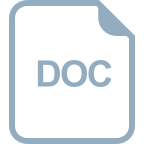
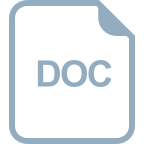

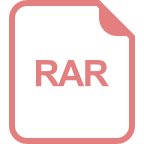
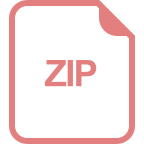
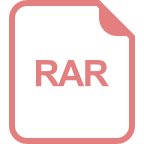
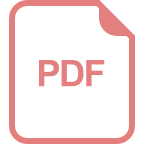
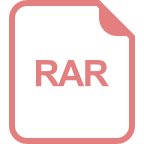






